Unit Testing Objective-C asychronous block-based API
March 10, 2014Unit Testing Objective-C block-based API
We are starting to utilize more Unit Testing for business logic and behavior design that’s going on under the hood in our apps.
Using Objective-C blocks for asynchronous operations in Apple’s Cocoa framework is an increasingly popular pattern. Built-in libraries such as Core Animation & Core Motion and 3rd-party libraries like AFNetworking utilize blocks extensively for asynchronous callbacks.
NSDictionary *params = @{@"id":@2}; [[MLStore sharedStore] GETWithParameters:params completionBlock:^void(id responseObject, NSError *err) { // do something with responseObject passed by block XCTAssert(responseObject != nil, @"GET response should not be nil."); }];
This will succeed every time because, most likely, the test case will have terminated by the time the block completes/returns to the caller. The test never fails because the Assert will never even be evaluated. So what’s the solution? Stack Overflow to the rescue!
My favorite (and simplest to understand, in my opinion) answer from that SO question is to use Grand Central Dispatch and the built-in semaphore constructs.
NSInterval TIMEOUT = 5.0; // create a semaphore with initial value of 0 dispatch_semaphore_t sem = dispatch_semaphore_create(0); [myConcurrentAPI doSomethingWithCompletion:^(id data, NSError *error) { // check error and do stuff with data dispatch_semaphore_signal(sem); // signal to OS to release/decrement semaphore }]; // check for the Semaphore signal but keep executing the main // run loop for TIMEOUT seconds while (dispatch_semaphore_wait(sem, DISPATCH_TIME_NOW)) { [[NSRunLoop currentRunLoop] runMode:NSDefaultRunLoopMode beforeDate:[NSDate dateWithTimeIntervalSinceNow:TIMEOUT]]; }
The program will execute the method with the aynchronous block, then stick at the while loop but continue executing the main run loop (i.e. not blocking execution of the main or other threads.) Once the block returns/completes, it signals the semaphore (a little bit of shared memory managed by the runtime/operating system) and your test method continues or completes.
If you need test multiple async calls in the same method, I recommend creating a new semaphore variable each time – don’t reuse an exisiting one.
Here are three C preprocessor macros that simplify the code a bit. You still need to provide a variable name but semicolons are optional.
#define SemaphoreSetup(SEM_NAME) dispatch_semaphore_t SEM_NAME = dispatch_semaphore_create(0); #define SemaphoreSignal(SEM_NAME) dispatch_semaphore_signal(SEM_NAME); #define SemaphoreWait(SEM_NAME) while (dispatch_semaphore_wait(SEM_NAME, DISPATCH_TIME_NOW)) { [[NSRunLoop currentRunLoop] runMode:NSDefaultRunLoopMode beforeDate:[NSDate dateWithTimeIntervalSinceNow:TIMEOUT]]; } /* Then use like this */ SemaphoreSetup(sem) [[MLStore sharedStore] GETWithParameters:params completionBlock:^void(id responseObject, NSError *err) { // do something with responseObject passed by block XCTAssert(responseObject != nil, @"GET response should not be nil."); SemaphoreSignal(sem) }]; SemaphoreWait(sem)
Happy Testing!
Looking for more like this?
Sign up for our monthly newsletter to receive helpful articles, case studies, and stories from our team.
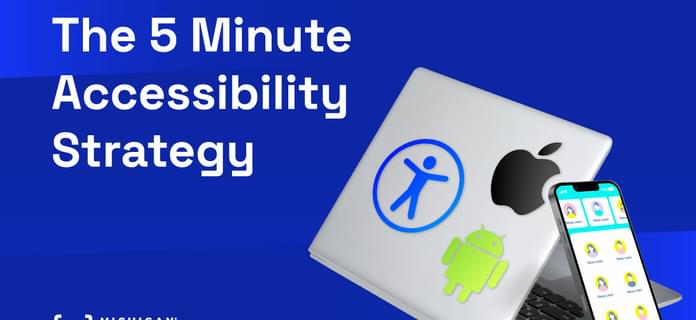
The 5 Minute Accessibility Strategy
May 18, 2023We discuss how you can make a plan in just 5 minutes to provide accessibility in your mobile app.
Read more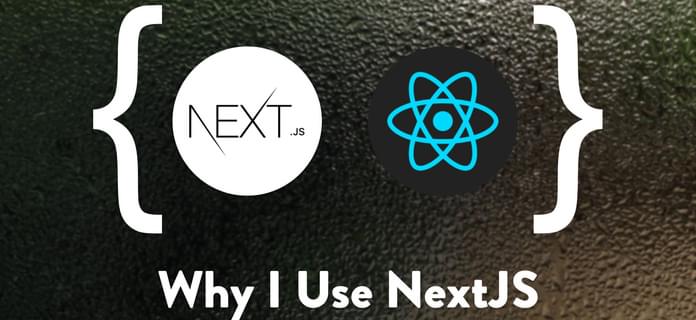
Why I use NextJS
December 21, 2022Is NextJS right for your next project? In this post, David discusses three core functionalities that NextJS excels at, so that you can make a well-informed decision on your project’s major framework.
Read more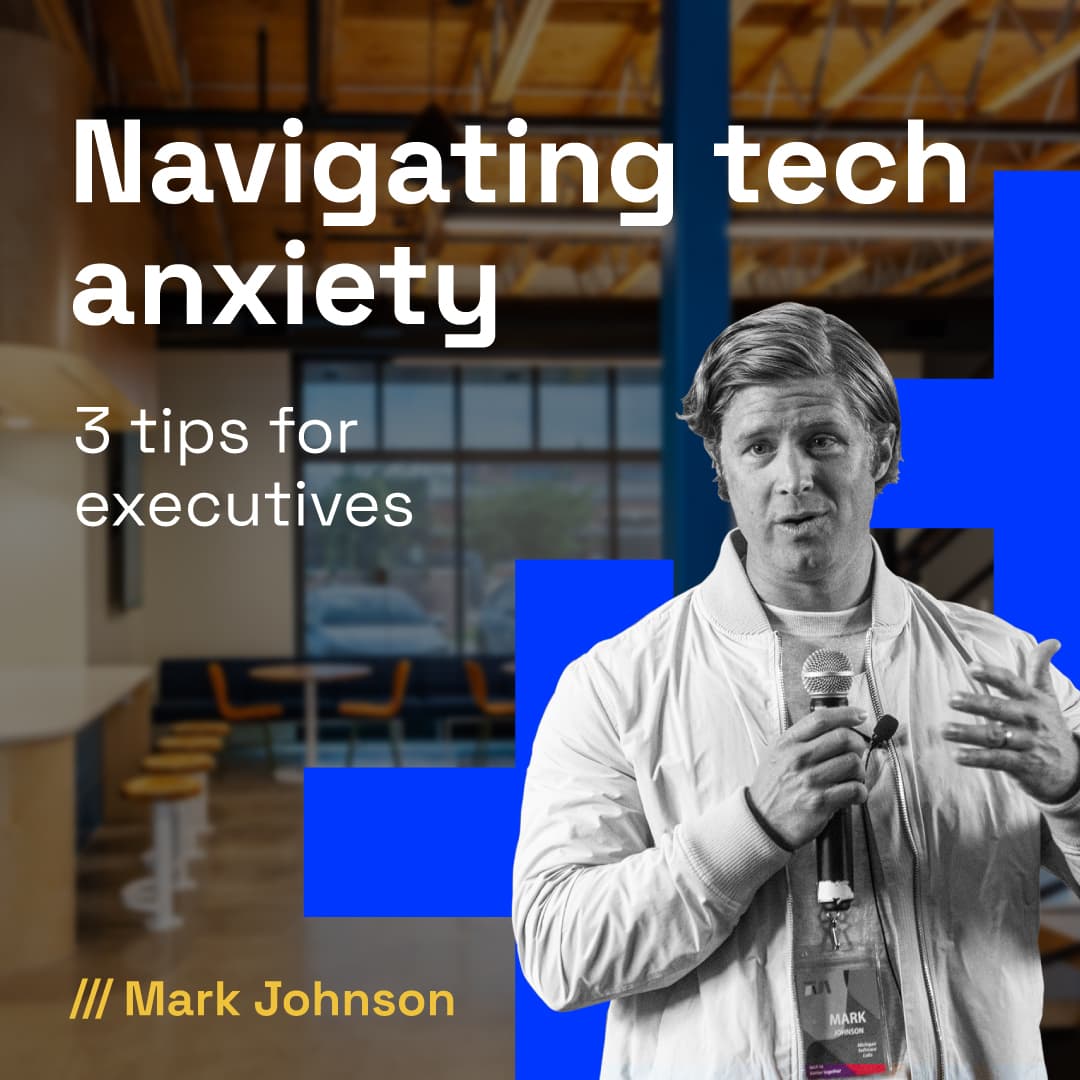
3 tips for navigating tech anxiety as an executive
March 13, 2024C-suite leaders feel the pressure to increase the tempo of their digital transformations, but feel anxiety from cybersecurity, artificial intelligence, and challenging economic, global, and political conditions. Discover how to work through this.
Read more