Using xibs/nibs in iOS 7 and Xcode 5
April 9, 2014We recently started a brand new project from scratch and decided to use Xcode’s NIB (abbreviation for NeXT Interface Builder) files to create and layout screens for the application’s user interface. Apple encourages using Storyboards, but we thought nib files would be less hassle with multiple developers and minimize merge conflicts due to version control software (e.g. git.) This debate could be the subject of a whole entire, separate blog post, but that’s for another day. I’ll just highlight some of what we’ve learned using Xcode 5 and nib files (that happen to use the .xib file extension, BTW.)
Create a new project:
So you want to start out your new project, but it seems like every iOS Application template uses Storyboards. Well you’re right, only the simple “Empty Application” does not include a Storyboard.
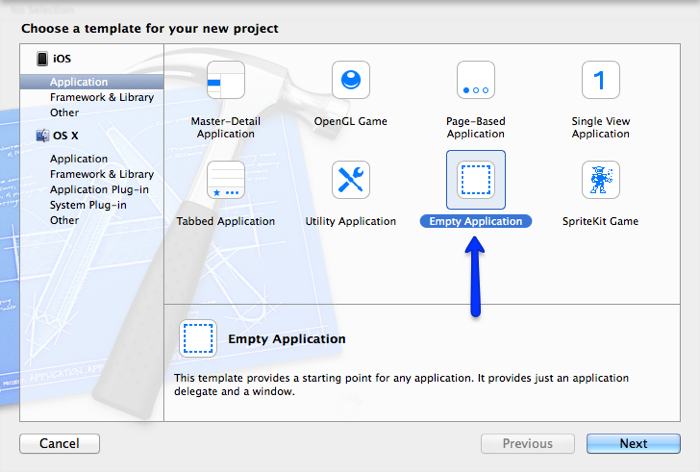
But this “Empty Application” is truly empty. There’s not even a view controller to present a view to the user. In fact, if you run the app you’ll likely get a warning: Application should have Root View Controller when ApplicationDidFinishLaunching
.
A common pattern is to create a RootViewController (with no view) to present and switch between other view controllers, in your AppDelegate.m:
self.window = [[UIWindow alloc] initWithFrame:[[UIScreen mainScreen] bounds]]; // This is code to create a view controller and set window's property UIViewController *rootViewController = [UIViewController new]; self.window.rootViewController = rootVC; // back to boilerplate [self.window makeKeyAndVisible]; return YES;
Manually present a new UIViewController
Easily create a new view controller with a nib file with File -> New -> Cocoa Touch -> Objective-C class and choose subclass of UIViewController and click checkbox “With XIB for user interface.”
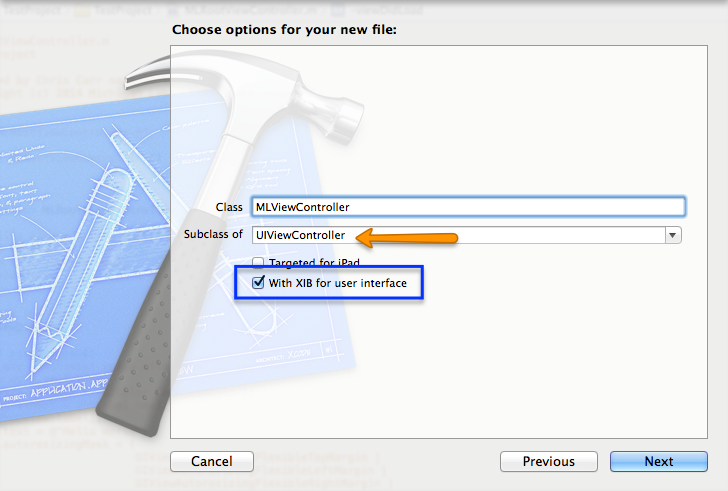
Xcode creates a xib file with the same name as your custom class. Another convenience is that Xcode will automatically load the view and objects contained in the nib when you instantiate the custom class – you can even remove the “Controller” part from end of the filename and the iOS runtime will still find and load the nib.
// in rootVC, create your next view controller and present it MLCustomViewController *nextVC = [MLCustomViewController new]; [self presentViewController:nextVC animated:YES completion:nil];
When you create a view controller this way, you’ll notice that the File’s Owner in the nib is set to a custom ViewController class and an IBOutlet is set from File’s Owner to the View top level object. This is how the iOS run-time can load the nib’s view automatically when the view controller is instantiated.
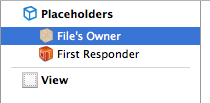
Create a ViewController with its view loaded from a custom NIB
When you need to instantiate a view controller with a nib defined in a variable at runtime, use this method:
NSString *aNibName = @"customNibName"; MyViewController* myViewController = [[MyViewController alloc] initWithNibName:aNibName bundle:nil]; [navigationController pushViewController:myViewController];
Load a NIB for a custom UIView subclass
There’s a couple of different ways to load a nib for a UIView (either a subclass or standard UIView.)
UIView *myView = [[[NSBundle mainBundle] loadNibNamed:@"MyViewNib" owner:self options:nil] lastObject]; MyViewClass *customView = [[[UINib nibWithNibName:@"MyCustomView" bundle:nil] instantiateWithOwner:self options:nil] objectAtIndex:0];
Both of these Nib loading methods return an NSArray with its top-level objects. Typically that just means the View (and all of the subviews it contains.) To be extra careful you can use runtime type checking with isKindOfClass:
. If you’ve created a UIView subclass, make sure to set the Custom Class in the Identity Inspector in Interface Builder. The File’s Owner placeholder object can be left blank (at the default, NSObject.) If you get the dreaded class is not key value coding-compliant for the key
Exception, it probably means you have an Outlet in the nib without a corresponding IBOutlet/Action in the class file.
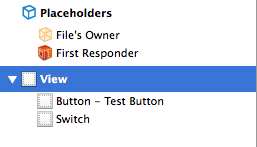
Customize UIView (or subclass) loaded from Nib
It is important to differentiate the object instantiation process when creating objects purely in code or loading them from a nib archive. Methods like initWithFrame:
and initWithStyle
are not called on a UIView subclass when creating it from a nib. Override initWithCoder:
instead.
- (id)initWithCoder:(NSCoder *)aDecoder { self = [super initWithCoder:aDecoder]; if (self) { // custom initialization here but no IBOutlets, yet } return self; }
One gotcha: IBOutlets are not created until AFTER initWithCoder:
is finished. In order to customize the objects loaded by the Nib, override awakeFromNib
.
- (void)awakeFromNib { self.myButton.layer.borderWidth = 1.0; self.switch.on = YES; }
There is lots more information available from Apple’s Developer website: Resource Management in View Controllers and Resource Programming Guide.
Looking for more like this?
Sign up for our monthly newsletter to receive helpful articles, case studies, and stories from our team.
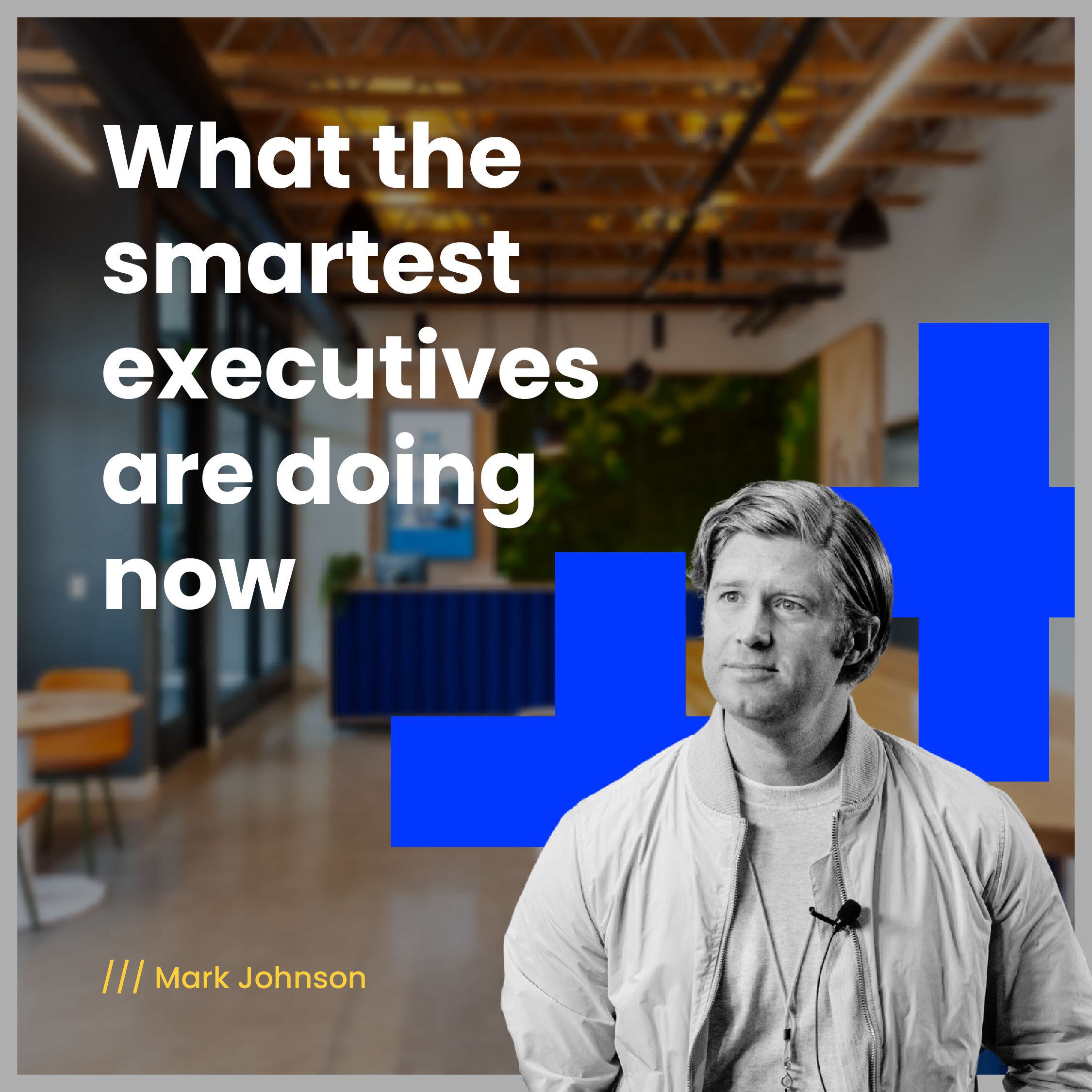
What the smartest executive leaders are doing right now
July 25, 2024We are consistently seeing three things right now in terms of how executives are tackling the technological challenges they are facing.
Read more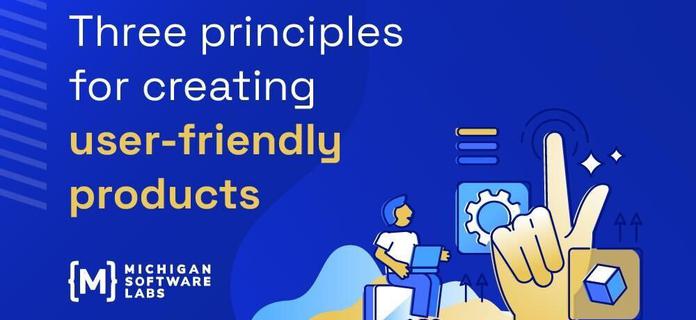
Three principles for creating user-friendly products
January 25, 2023Grayson discusses three guiding principles he utilizes when designing user experiences for products.
Read more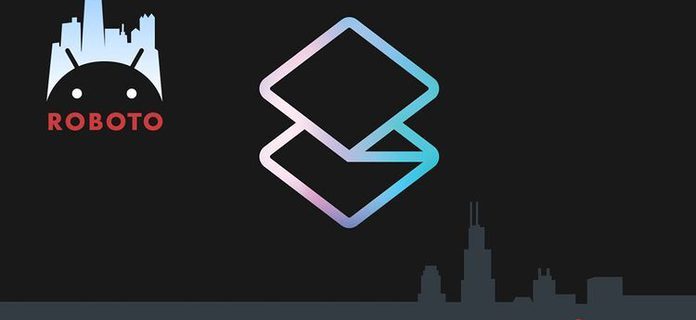
Chicago Roboto 2022 Retrospective
August 11, 2022Scott Schmitz shares some notes of interest from talks at Chicago Roboto 2022, an Android community conference, that took place August 1-2.
Read more