Why isn’t my Fragment showing? A quirk of FragmentTransactions
March 26, 2015I stumbled upon an interesting situation while working on an Android project. I was trying to display two different Fragments at the same time, but one of them wasn’t showing up and I wasn’t getting any feedback as to why it wasn’t showing up. I was also unable to find any documentation that might explain why it was happening.
In the Android ecosystem, a Fragment is a self-contained portion of the user interface, which may or may not have graphical components. For those Fragments that do have graphical components, they can be inserted into a View in an Activity using a FragmentTransaction. A FragmentTransaction keeps track of one or many add, remove, or replace operations you want to perform in a single transaction.
An interesting quirk comes into play if multiple operations are performed in a single FragmentTransaction. Let’s say you have two fragments, FragmentA and FragmentB. Let’s add FragmentA to fragmentContainerView1, a View in an Activity:
getSupportFragmentManager().beginTransaction() .add(R.id.fragmentContainerView1, fragmentA) .commit();
Later on, we want to perform a transaction where FragmentA is placed in fragmentContainerView1 and FragmentB is placed in fragmentContainerView2. However, FragmentA may or may not still be in fragmentContainerView1.
Let’s attempt to perform this operation:
getSupportFragmentManager().beginTransaction() .replace(R.id.fragmentContainerView1, fragmentA) .replace(R.id.fragmentContainerView2, fragmentB) .commit();
If FragmentA weren’t currently in fragmentContainerView1, then this operation will proceed as expected and both Fragments will be placed in their respective Views. However, if FragmentA is currently in fragmentContainerView1 then the first replace() operation wouldn’t go through since FragmentA is already there. What will also happen is that the replace() operation for FragmentB will not go through. There isn’t any warning or indication that FragmentB’s replace() operation did not go through other than that it won’t show up in the expected View.
To prevent this from happening, simply check if the fragment has been added yet or not, and if not, then perform the replace, like so:
FragmentTransaction fragmentTransaction = getSupportFragmentManager().beginTransaction(); if (!fragmentA.isAdded()) { fragmentTransaction.replace(R.id.fragmentContainerView1, fragmentA); } if (!fragmentB.isAdded()) { fragmentTransaction.replace(R.id.fragmentContainerView2, fragmentB); } fragmentTransaction.commit();
If you are experiencing a problem similar to this, you might just be encountering a situation where one of the operations in your FragmentTransaction is failing and is causing the other operations to fail as well.
Looking for more like this?
Sign up for our monthly newsletter to receive helpful articles, case studies, and stories from our team.
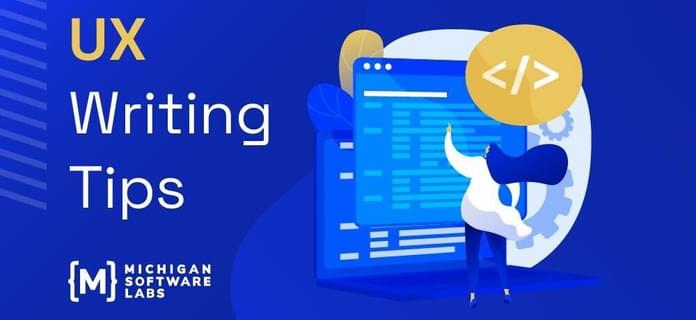
UX Writing Tips
February 3, 2023Kai shares a few tips he's collected on how to write for user interfaces.
Read more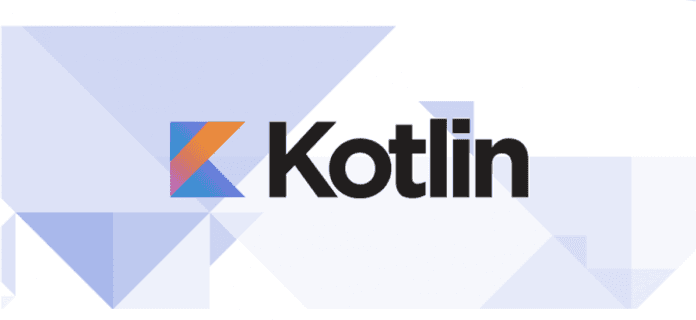
Kotlin Multiplatform
July 14, 2022A brief look at Kotlin Multiplatform Mobile, a newer cross-platform mobile application framework.
Read more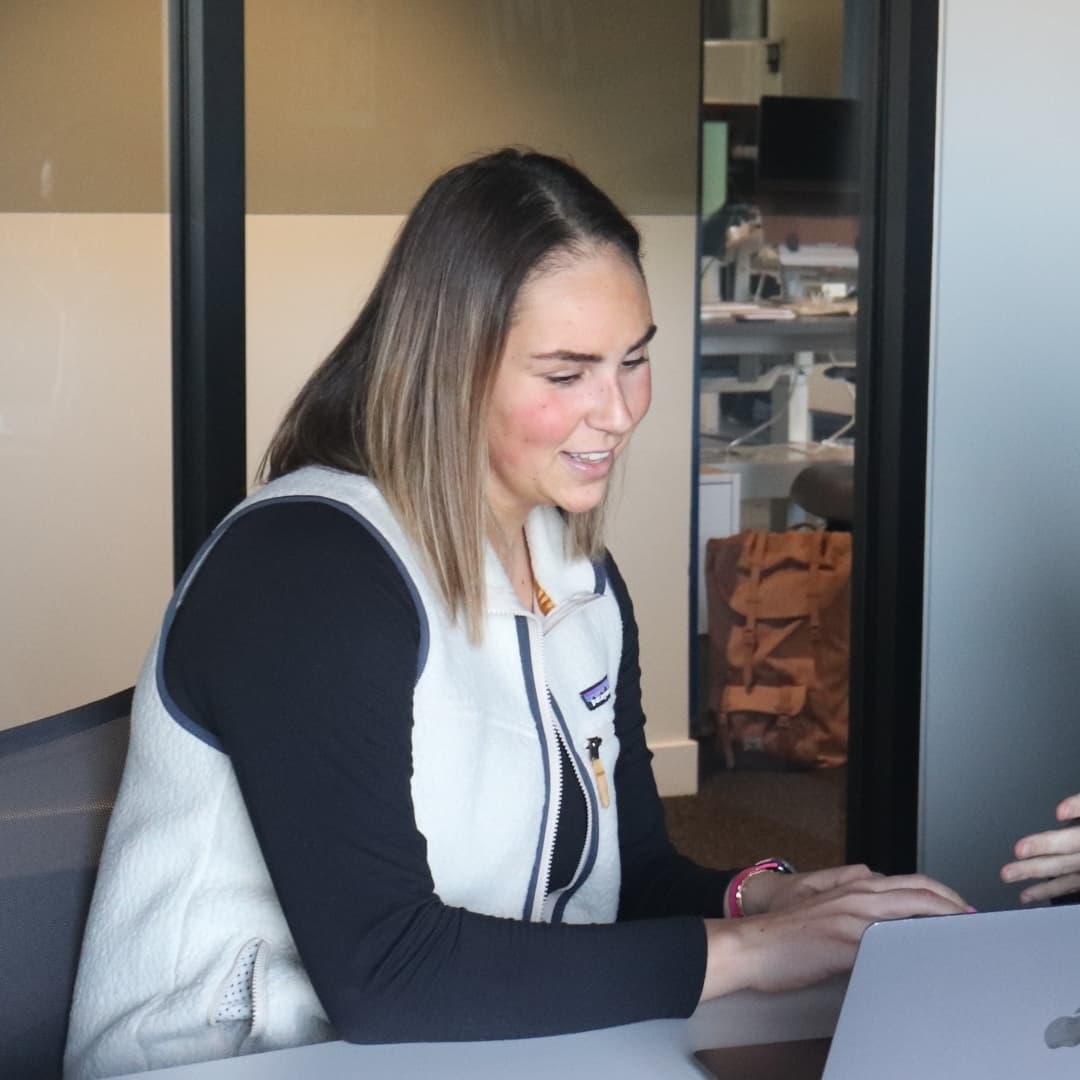
Tech is for everyone
June 20, 2024Have you ever felt like the tech world was an Ivy league school, where only the most elite students gain acceptance? Discover paths into the industry you may not have considered.
Read more