Reusing Custom Views in Xcode
June 29, 2015We recently started work on an app that reuses similar components across various screens. In an effort to be good stewards of the project, we decided to create a reusable view which could then be added to a container view. This proved to be a bit trickier than I had hoped, but the solution we came up with provides some nice flexibility.
For the purposes of this blog post we will be building a app that looks like the mockup below.
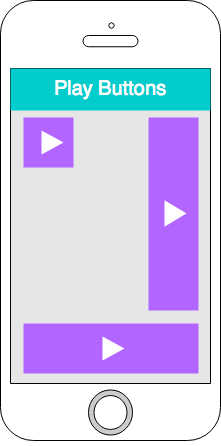
Designing Your View
Our current process is to design all of our views (with a few exceptions) using Interface Builder and XIB files. To accomplish our goal of reusing this play button view, we will need to create two different XIB files. First we will create the container view that will hold the reusable play buttons. The XIB will look something like the image below.
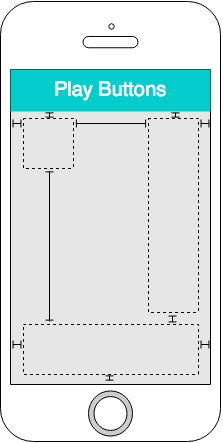
Note that we are constraining what will be our container views to the margins of the View Controller’s view (their super view). From here the constraints we put on the subview will take over. This approach lets us Modularize our UI into these separate components.
Designing Your Subview
Designing and constraining a custom subview requires a little extra thought in how you set up its constraints. The subview does not know what size container it might be put in, and as such you need to constrain it so that it looks good in all (reasonable) containers.
Below is a mockup of the constraints our custom, reusable play button might have.
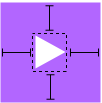
Based on our mock up, the play button should expand the play icon to fit in the container while maintaining aspect ratio and a minimum margin between the edge of the icon and the edge of the button. Below is what this looks like in Interface Builder.
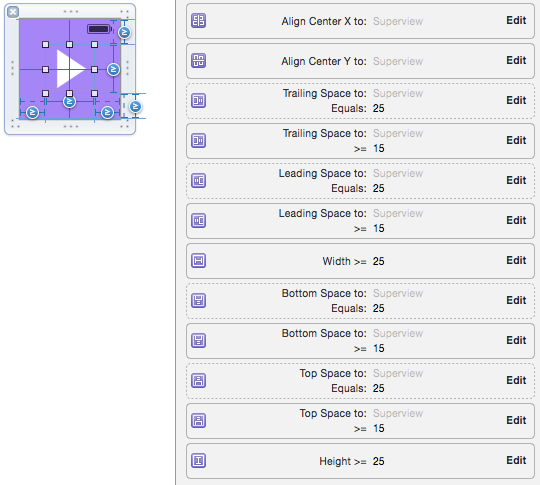
Now that we have our subview all laid out and constrained, we can add it to the container view we created earlier.
Putting them Together
This is where things get a little tricky. Simply adding our custom play button as a subview to the containers we set up earlier will not constrain the button to its container. As a result, we need to do that manually using a custom class for the container in Interface Builder. Create a new class called something like MLContainerView
. All this class needs to do is override the didAddSubview:
method with the following.
#import "MLContainerView.h" @implementation MLContainerView - (void)didAddSubview:(UIView *)subview { [super didAddSubview:subview]; [subview setTranslatesAutoresizingMaskIntoConstraints:NO]; NSDictionary *views = @{ @"sub" : subview }; NSMutableArray *constraints = [NSMutableArray new]; [constraints addObjectsFromArray: [NSLayoutConstraint constraintsWithVisualFormat:@"H:|[sub]|" options:NSLayoutFormatDirectionLeadingToTrailing metrics:nil views:views]]; [constraints addObjectsFromArray: [NSLayoutConstraint constraintsWithVisualFormat:@"V:|[sub]|" options:NSLayoutFormatDirectionLeadingToTrailing metrics:nil views:views]]; [self addConstraints:constraints]; } @end
What this method does is pin the added subview to the horizontal and vertical edges of the container. From there the auto-layout constraints of the play button will take over to layout our reusable view.
Putting all of this together we end up with an app that closely resembles our original mock up, while making maintenance of our view easier. By containing components of the UI in a separate layout, the container and the button layouts can change independently of one another, easing the headache of having multiple developers work on the same screen.
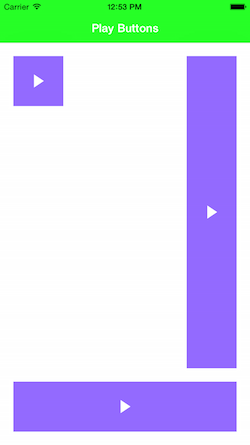
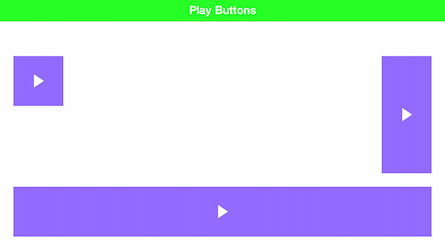
The final product for this demonstration can be found on github.
Looking for more like this?
Sign up for our monthly newsletter to receive helpful articles, case studies, and stories from our team.
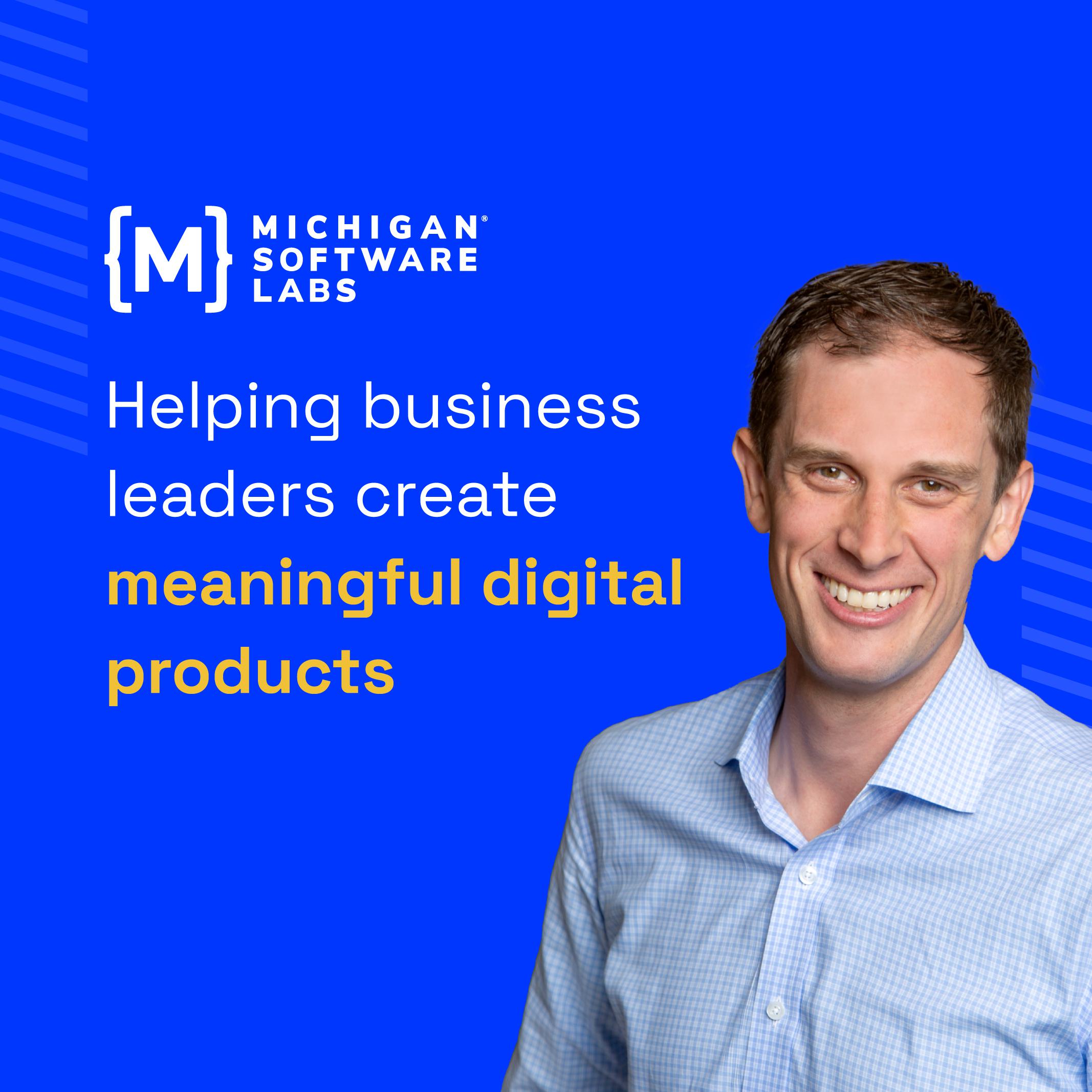
Business Model Canvas: Helping business leaders create meaningful digital products
January 17, 2024When it comes to custom software, it’s easy to feel stuck between needing to deliver value quickly, while also ensuring meaningful, long-lasting results. Learn why the Business Model Canvas can help you identify the right digital product for your business needs.
Read more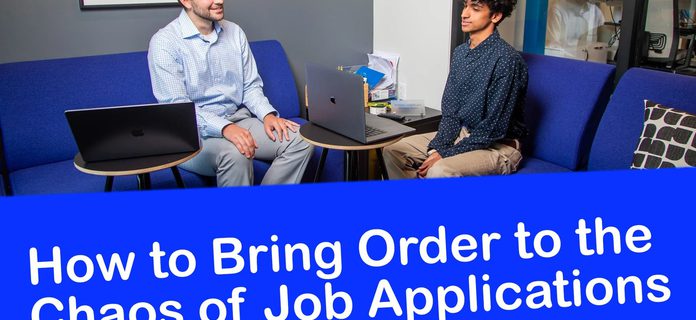
How to Bring Order to the Chaos of Job Applications
August 8, 2023We discuss some tips & tricks for the job application process
Read more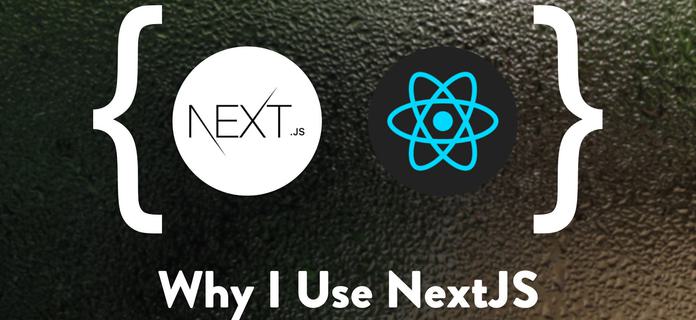
Why I use NextJS
December 21, 2022Is NextJS right for your next project? In this post, David discusses three core functionalities that NextJS excels at, so that you can make a well-informed decision on your project’s major framework.
Read more