Using the GPS in an Android App
August 14, 2014Accessing and getting an Android device’s current location has a few important steps.
Include the Google Play Services library
The LocationClient class from the Google Play Services library takes care of a lot of the meticulous work of detecting and managing the many ways that an Android device can determine its GPS location. This simplifies the work of the developer.
If you’re using Gradle to manage your project’s dependencies, add this to the “dependencies” block:
dependencies { compile 'com.google.android.gms:play-services:5.0.+' }
Now you can import all the needed location classes with:
import android.location.Location; import com.google.android.gms.common.ConnectionResult; import com.google.android.gms.common.GooglePlayServicesClient.ConnectionCallbacks; import com.google.android.gms.common.GooglePlayServicesClient.OnConnectionFailedListener; import com.google.android.gms.location.LocationClient; import com.google.android.gms.location.LocationListener; import com.google.android.gms.location.LocationRequest;
Implementing the Location Callbacks in your Activity
Implement the following classes in the Activity (or Fragment) that you want to use location services:
public class LocationExampleActivity extends Activity implements ConnectionCallbacks, OnConnectionFailedListener, LocationListener {
This requires you to implement the following methods:
// for ConnectionCallbacks @Override public void onConnected(Bundle bundle) { } // for ConnectionCallbacks @Override public void onDisconnected() { } // for OnConnectionFailedListener @Override public void onConnectionFailed(ConnectionResult connectionResult) { } // for LocationListener @Override public void onLocationChanged(Location location) { }
LocationClient
LocationClient is the main mechanism to retrieve the device’s GPS location. Add and create a LocationClient and a LocationRequest.
private LocationClient locationClient; private LocationRequest locationRequest; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); locationClient = new LocationClient(this, this, this); locationRequest = LocationRequest.create() .setPriority(LocationRequest.PRIORITY_HIGH_ACCURACY) .setInterval(1000) .setFastestInterval(1000); }
The LocationClient now uses our LocationExampleActivity for its listeners
The LocationRequest is how you define settings like how much positional accuracy you need versus how much power you want the GPS to draw, and at what frequency you want to query the GPS for your location.
(You may experience problems with your location not being acquired if you use a priority other than LocationRequest.PRIORITY_HIGH_ACCURACY, due to a bug.)
Connecting and Disconnecting
Initiate the connection to location services with:
@Override public void onStart() { super.onStart(); locationClient.connect(); } @Override public void onConnected(Bundle bundle) { Log.i(TAG, "locationClient connected"); if (locationClient != null) { locationClient.requestLocationUpdates(locationRequest, this); } } @Override public void onDisconnected() { Log.i(TAG, "locationClient disconnected"); } @Override public void onStop() { if (locationClient != null) { if (locationClient.isConnected()) { locationClient.removeLocationUpdates(this); locationClient.disconnect(); } } super.onStop(); }
Initiate the connection (in this case, in the Activity’s onStart() call). When onConnected() is called, you are clear to begin requesting the device’s location.
When the Activity is stopped and onStop() is called, we perform the clean up of location services by removing location updates and disconnecting from location services.
If location services are not available for a device
If a device does not have location services available, Google provides a way to prompt the user to go out and download Google Play Services.
public final static int CONNECTION_FAILURE_RESOLUTION_REQUEST = 9000; @Override public void onConnectionFailed(ConnectionResult connectionResult) { Log.i(TAG, "locationClient connection failed"); if (connectionResult.hasResolution()) { try { connectionResult.startResolutionForResult( this, CONNECTION_FAILURE_RESOLUTION_REQUEST); } catch (IntentSender.SendIntentException e) { Log.e(TAG, "IntentSender.SendIntentException", e); } } else { Log.e(TAG, "connectionResult has no resolution"); } } @Override public void onActivityResult(int requestCode, int resultCode, Intent data) { switch (requestCode) { case CONNECTION_FAILURE_RESOLUTION_REQUEST: switch (resultCode) { case Activity.RESULT_OK: locationClient.connect(); break; } } }
Getting the device’s location
Finally, after all that setup, we can start receiving location updates.
@Override public void onLocationChanged(Location location) { Log.i(TAG, "Location updated: " + location.getLatitude() + ", " + location.getLongitude()); // do something with the Location }
Now you can get the GPS latitude and longitude of the device’s current location.
A Github gist of the complete LocationExampleActivity.java can be found here.
Looking for more like this?
Sign up for our monthly newsletter to receive helpful articles, case studies, and stories from our team.
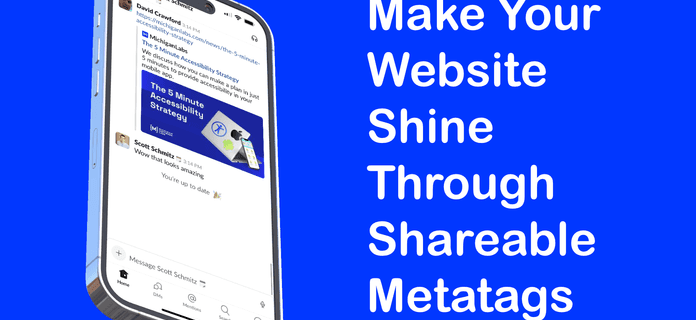
Make Your Website Shine Through Shareable Meta tags
June 20, 2023Improve the web site preview used by chat apps, websites, and social media with these easy tips.
Read more