BLOG
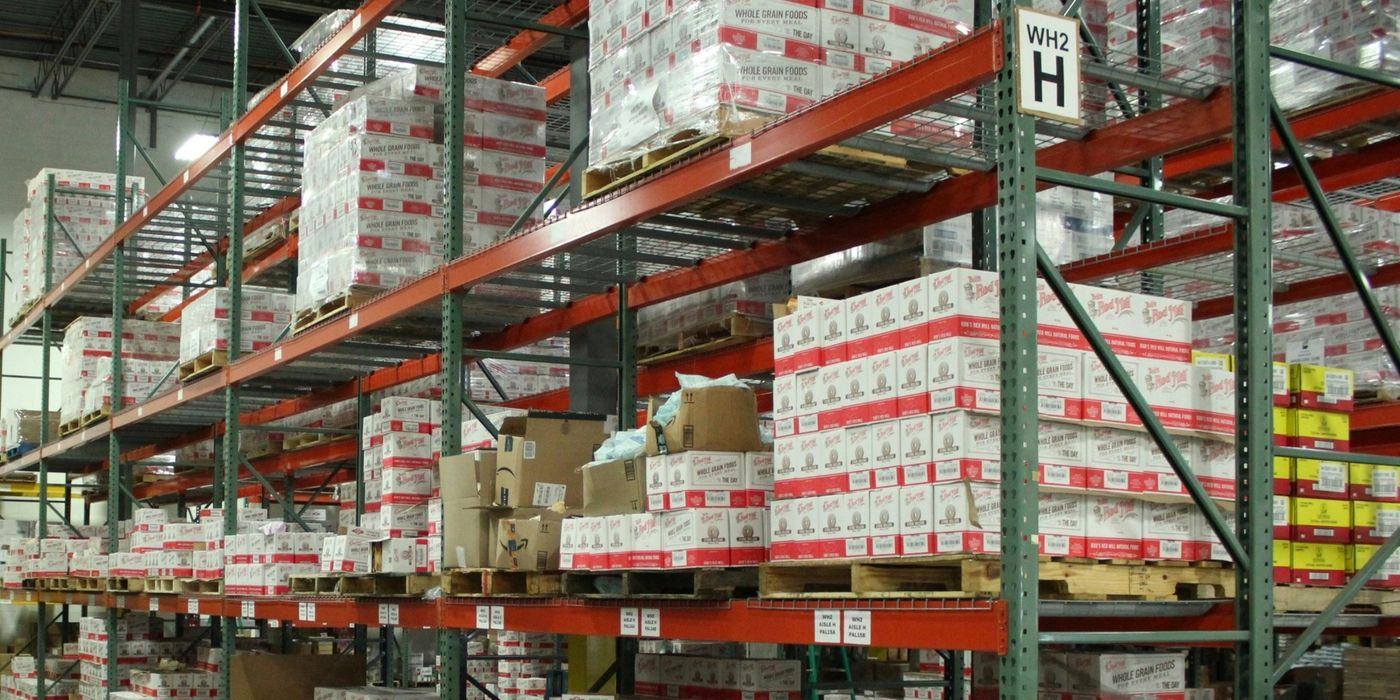
Can you trust AI to forecast your inventory? Here’s how to get it right
Learn how to build a trustworthy AI inventory forecasting system—with explainability, clear visuals, and tools your team can actually use.
Trending topics:
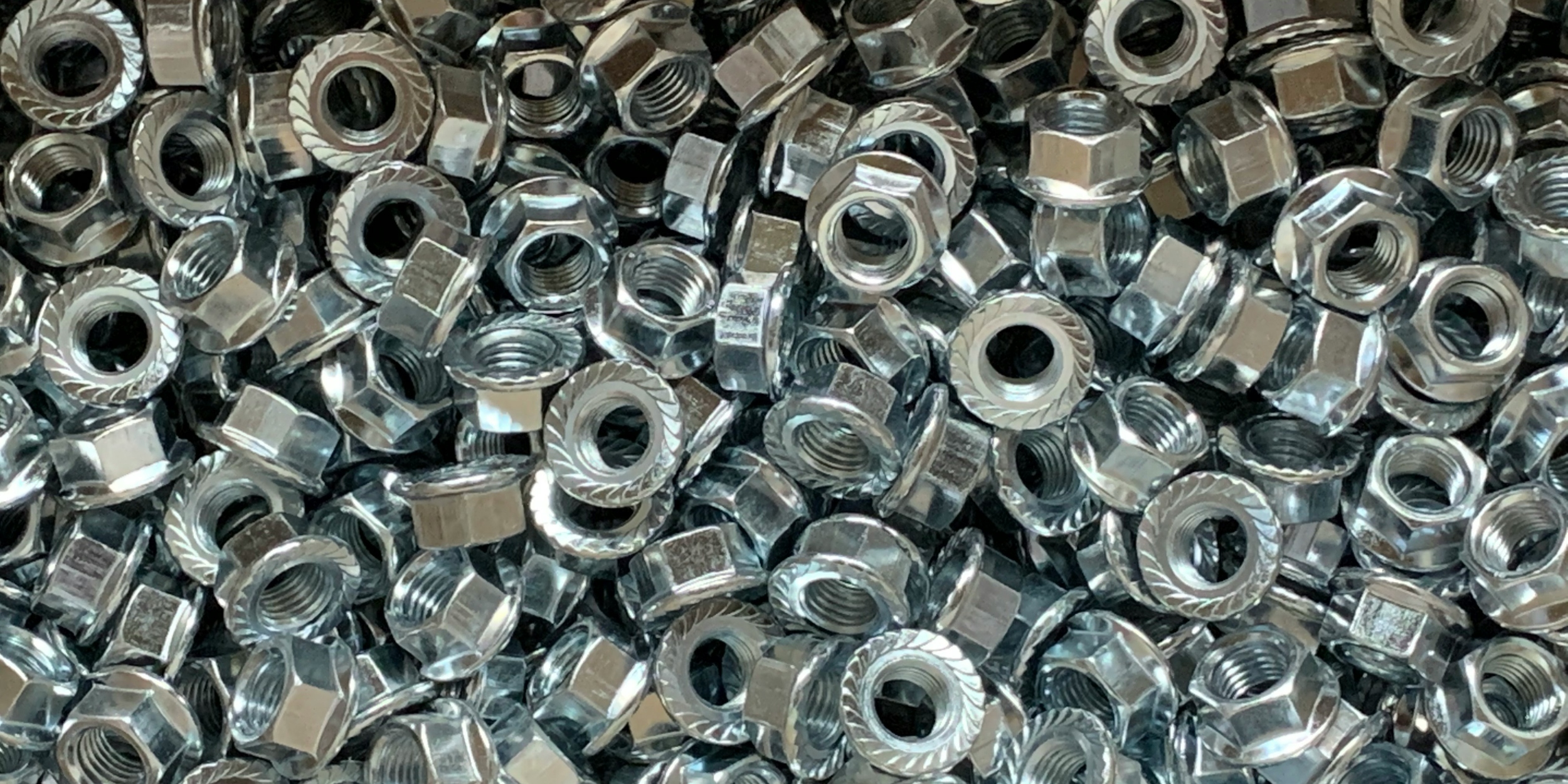
Explore how a quick computer vision prototype can validate defect detection in manufacturing: saving time, reducing waste, and de-risking automation.
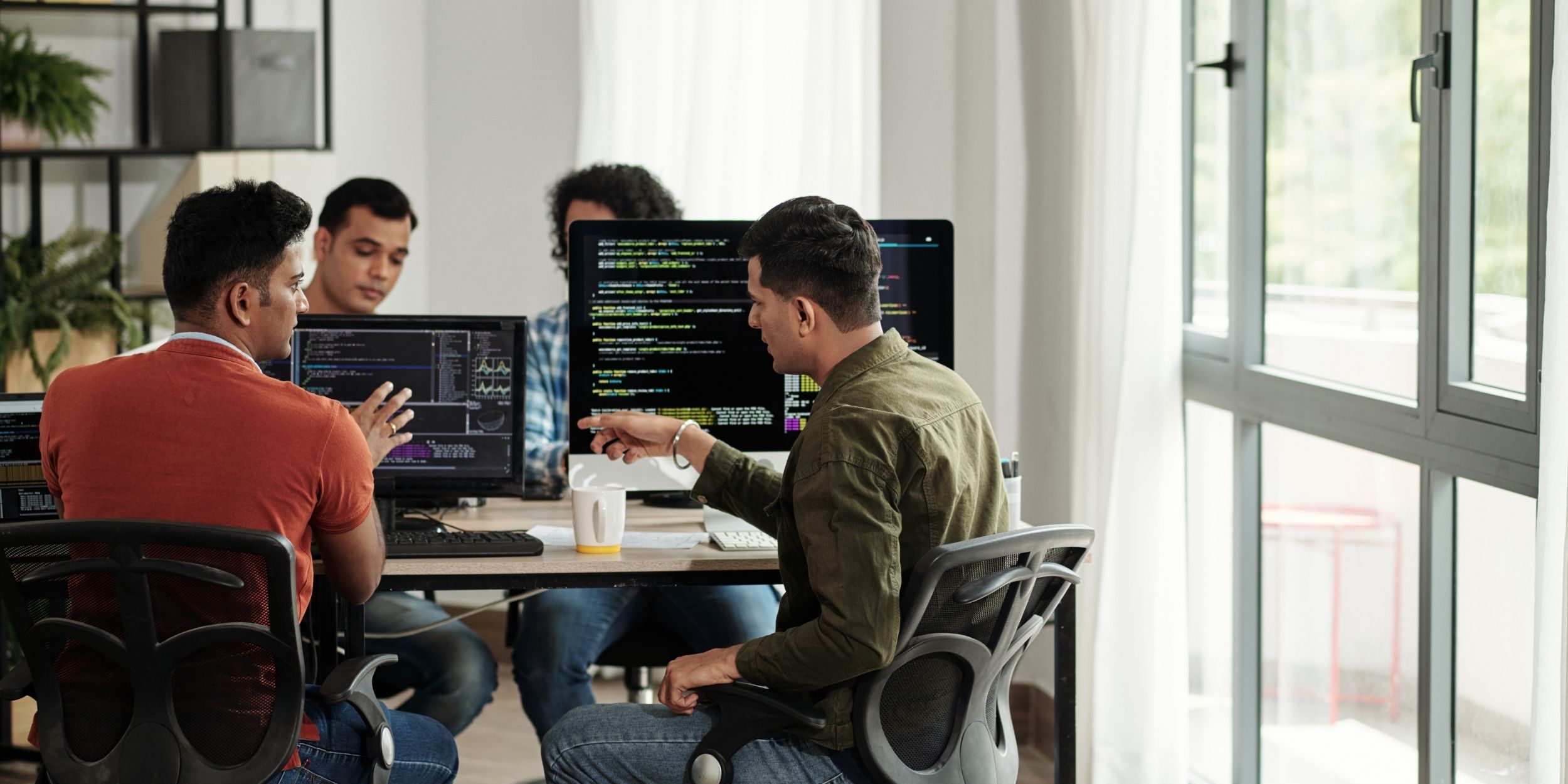
Optimize software ROI by making smart tech stack choices that boost performance, scalability, and cost-efficiency for long-term success
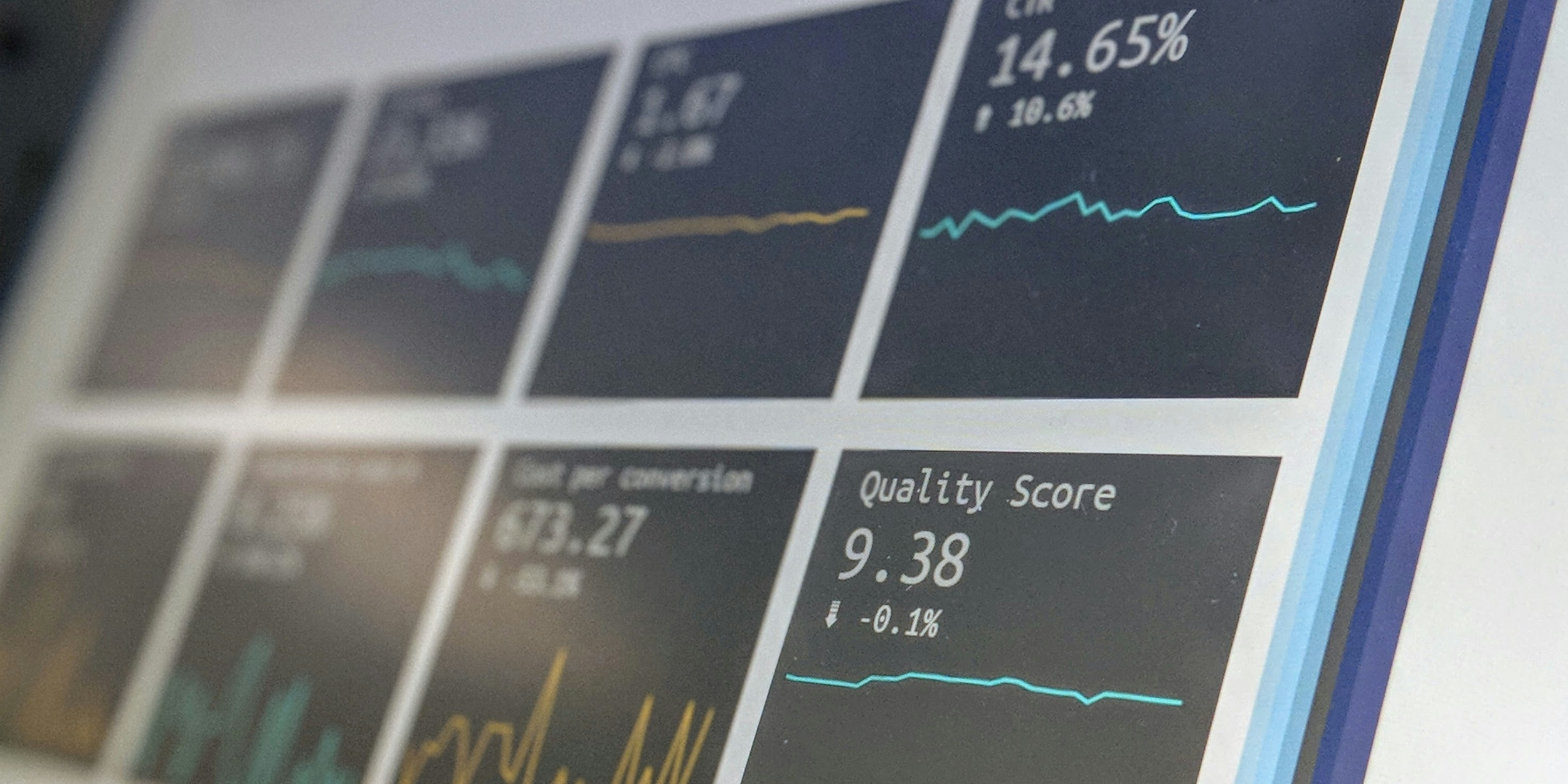
Is your company ready for AI? Discover why data maturity is the critical first step. Learn practical ways to assess and improve your data and explore the role of cloud infrastructure.
Smart takes and real stories from the digital product world
Choose the content that fits your focus. Whether you're shaping digital strategy, leading a team, or just want to stay connected—our newsletters are built to meet you where you are.
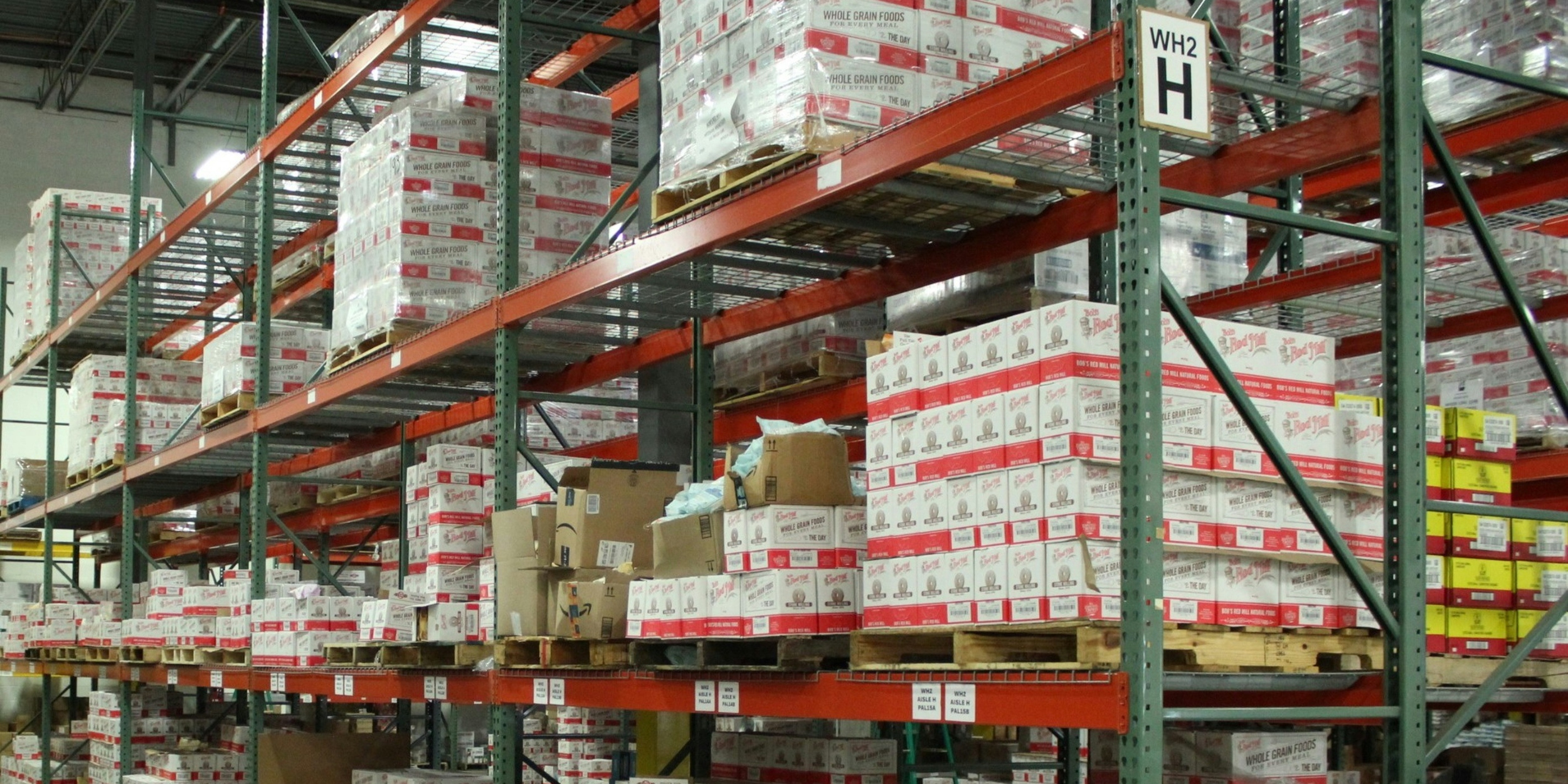
Learn how to build a trustworthy AI inventory forecasting system—with explainability, clear visuals, and tools your team can actually use.
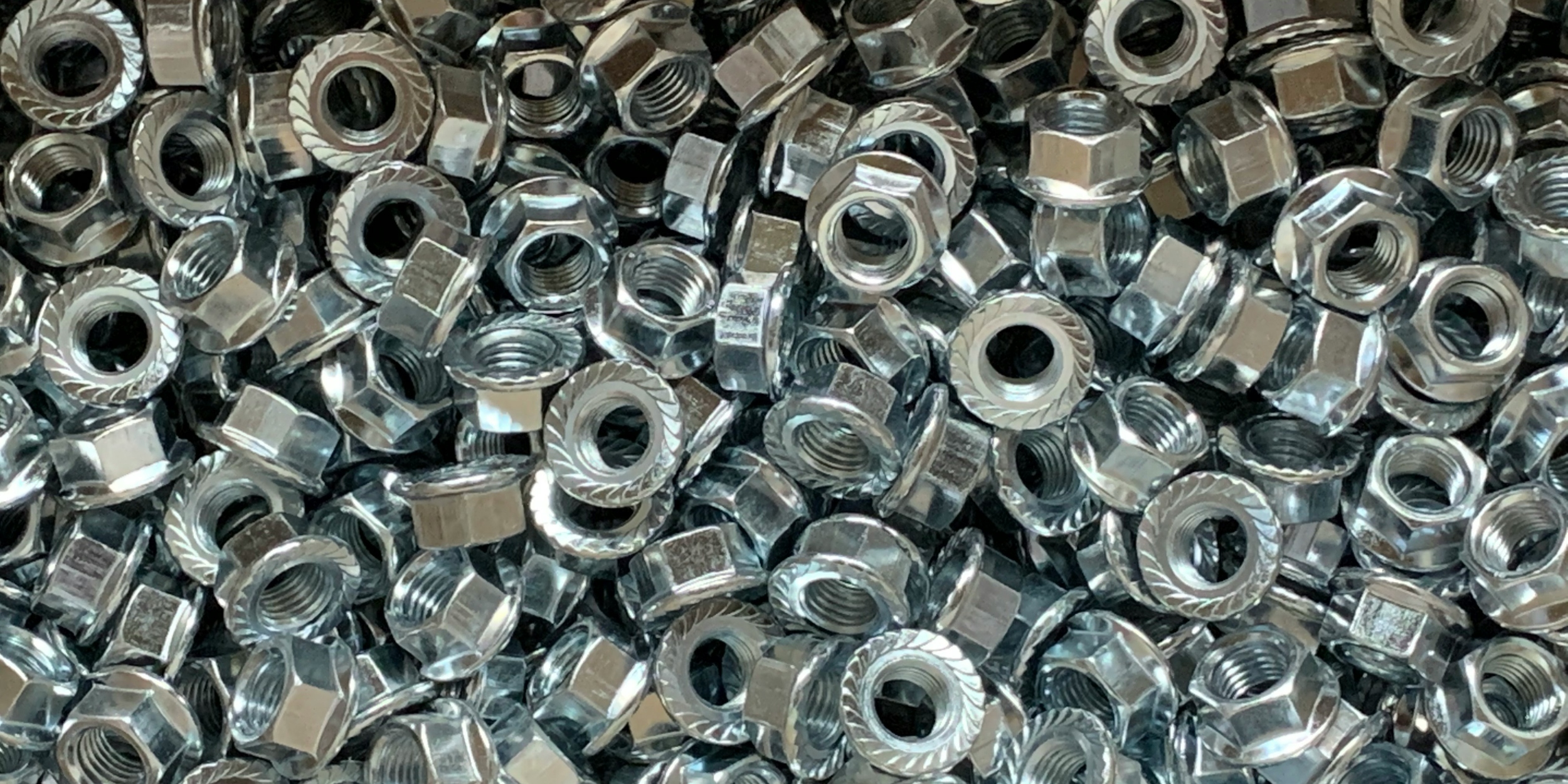
Explore how a quick computer vision prototype can validate defect detection in manufacturing: saving time, reducing waste, and de-risking automation.
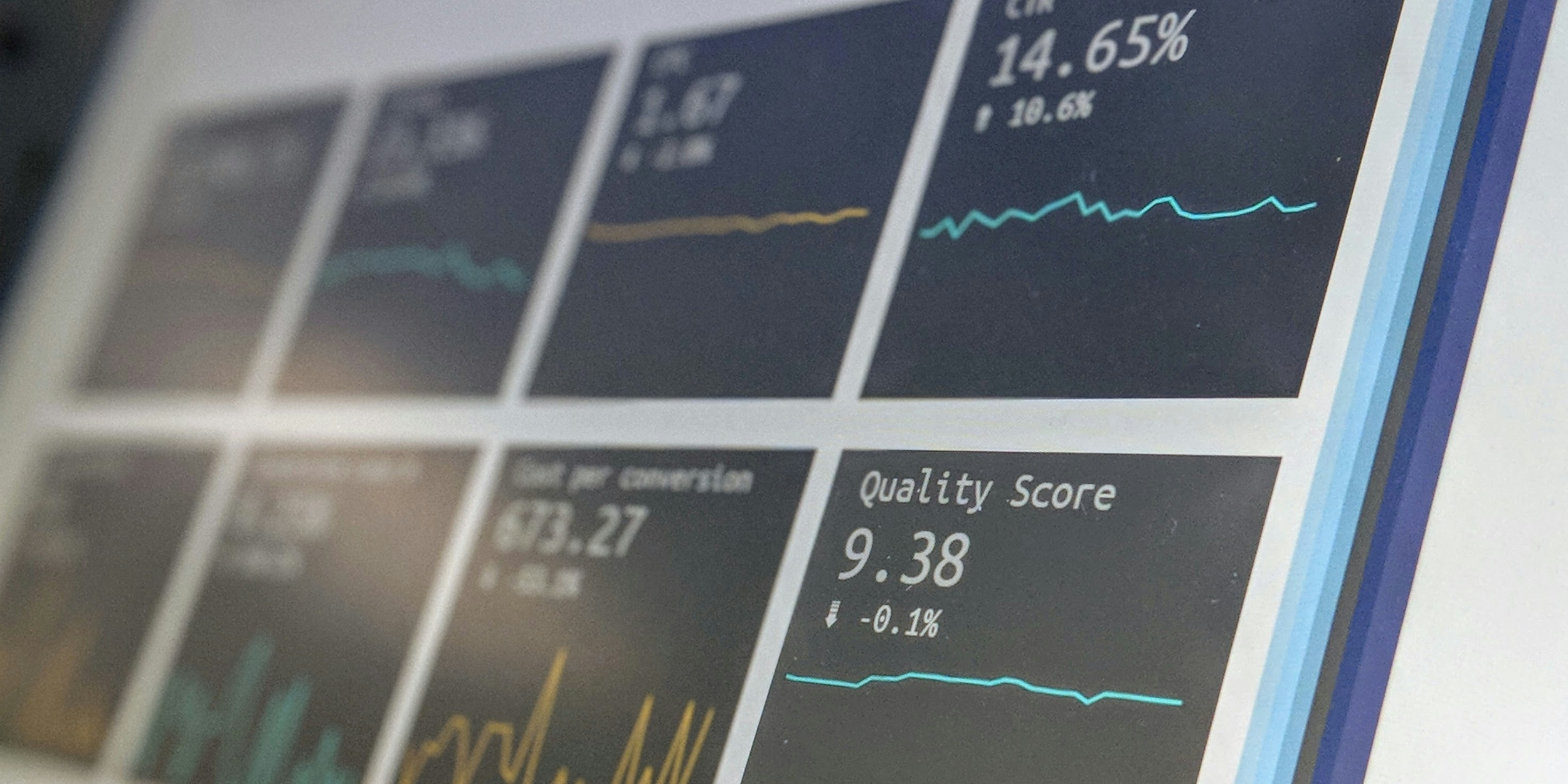
Is your company ready for AI? Discover why data maturity is the critical first step. Learn practical ways to assess and improve your data and explore the role of cloud infrastructure.
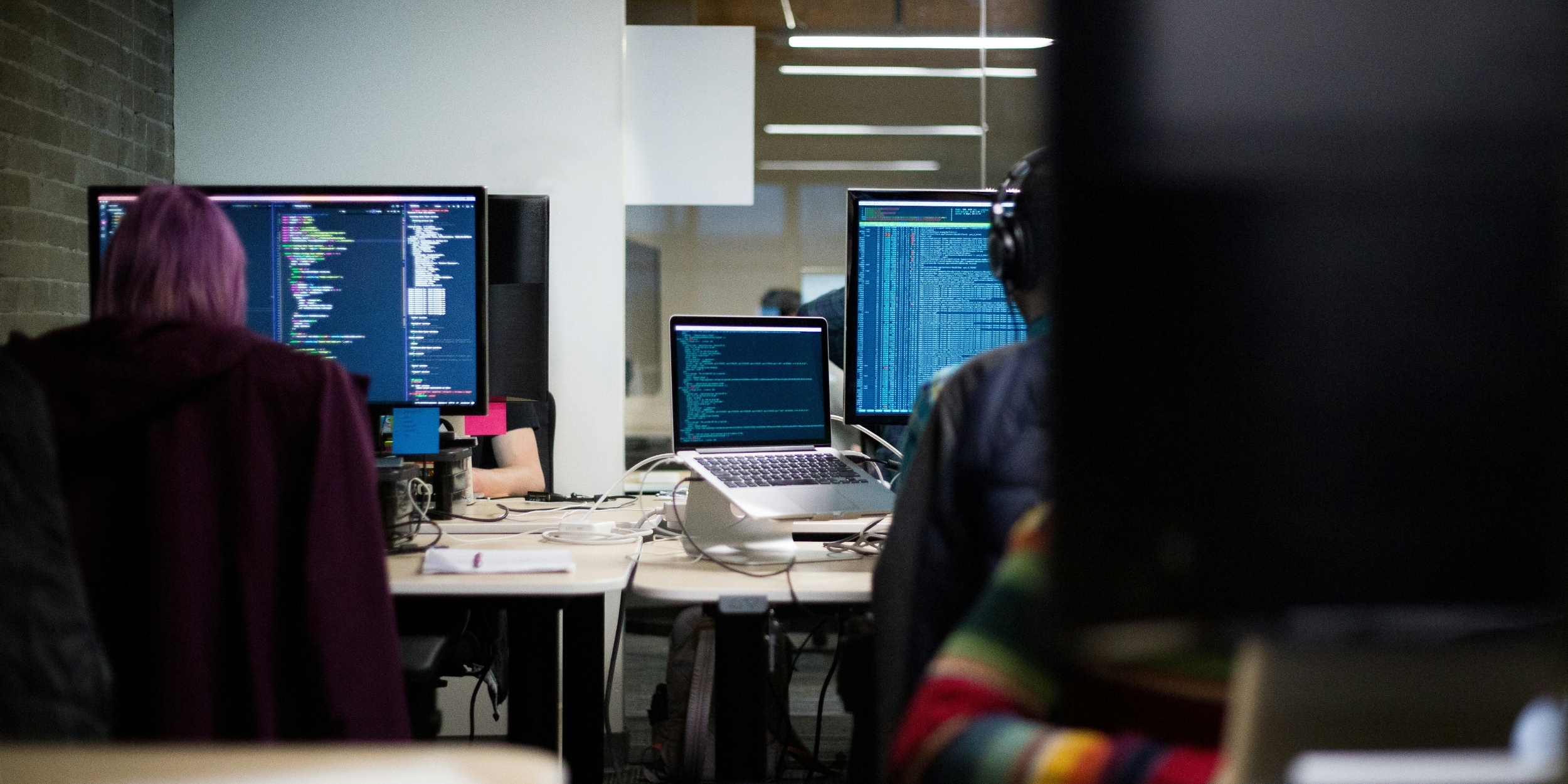
Explore the journey of Gradle and build tools like it, particularly in the context of Android development.
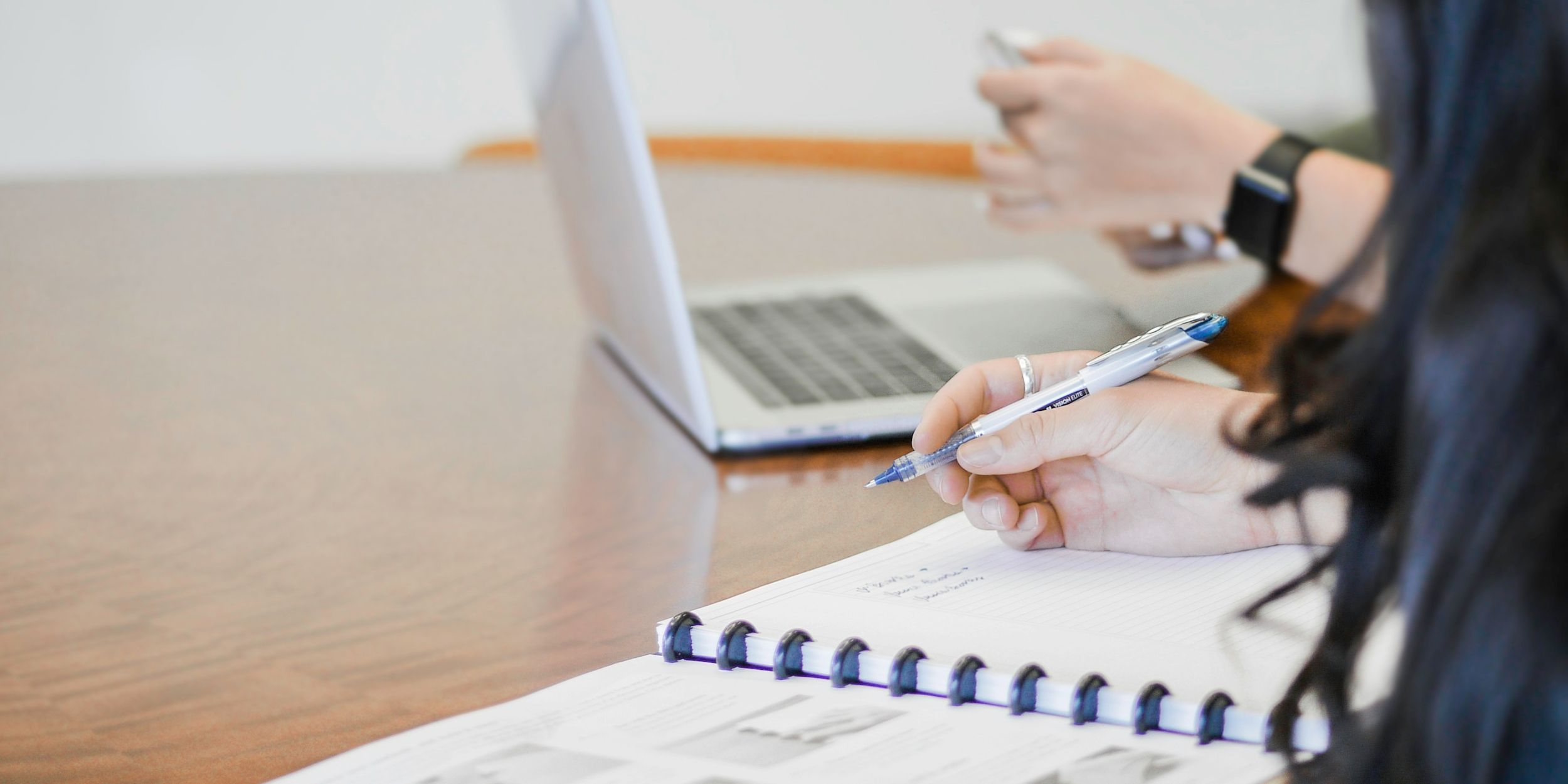
Can you clearly articulate your business strategy? Learn why the Business Model Canvas is a strong tool to deliver meaningful custom software products
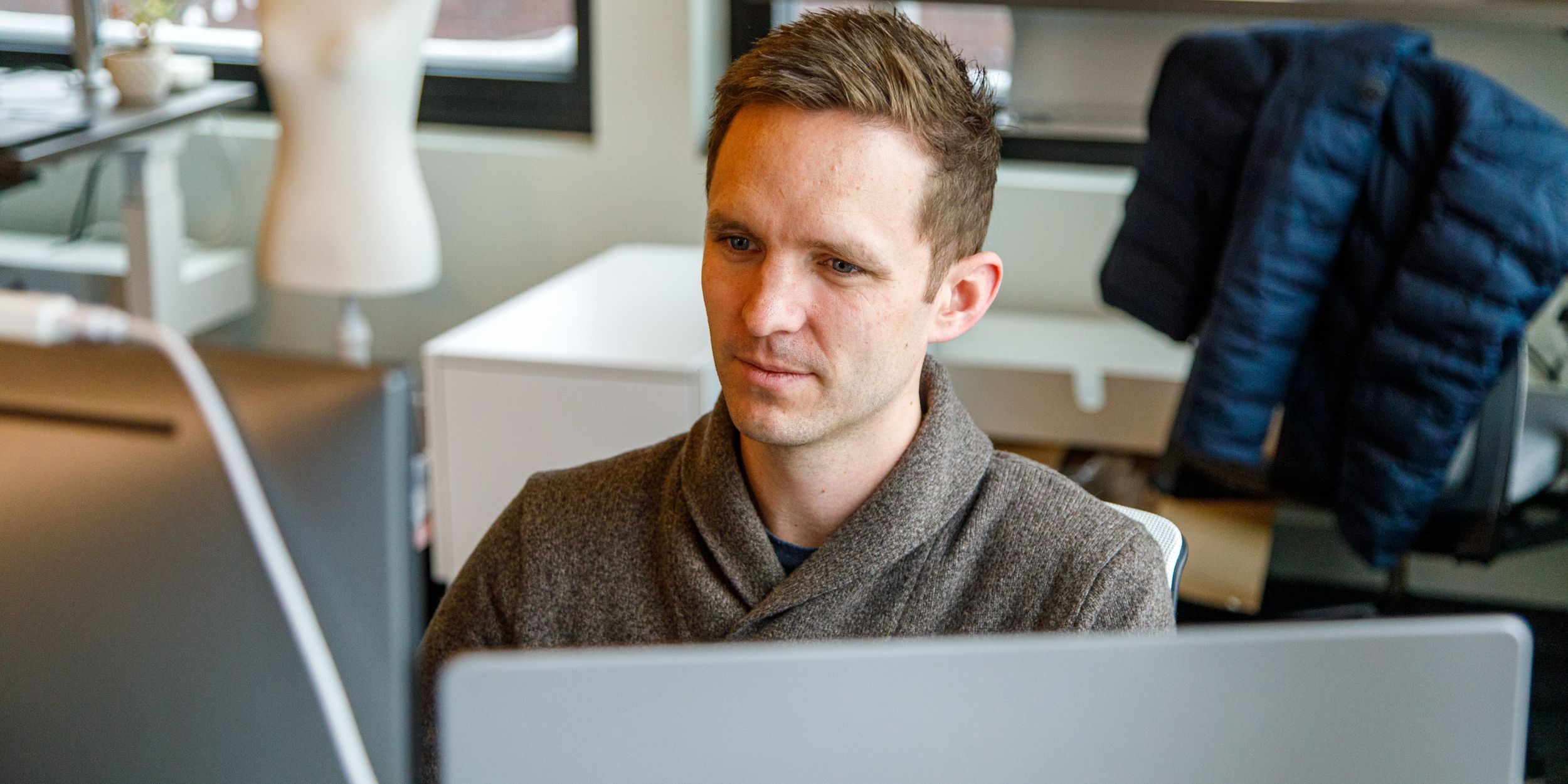
Custom software development is about crafting digital products that solve real user problems and deliver tangible business value. Development is more than just writing code.
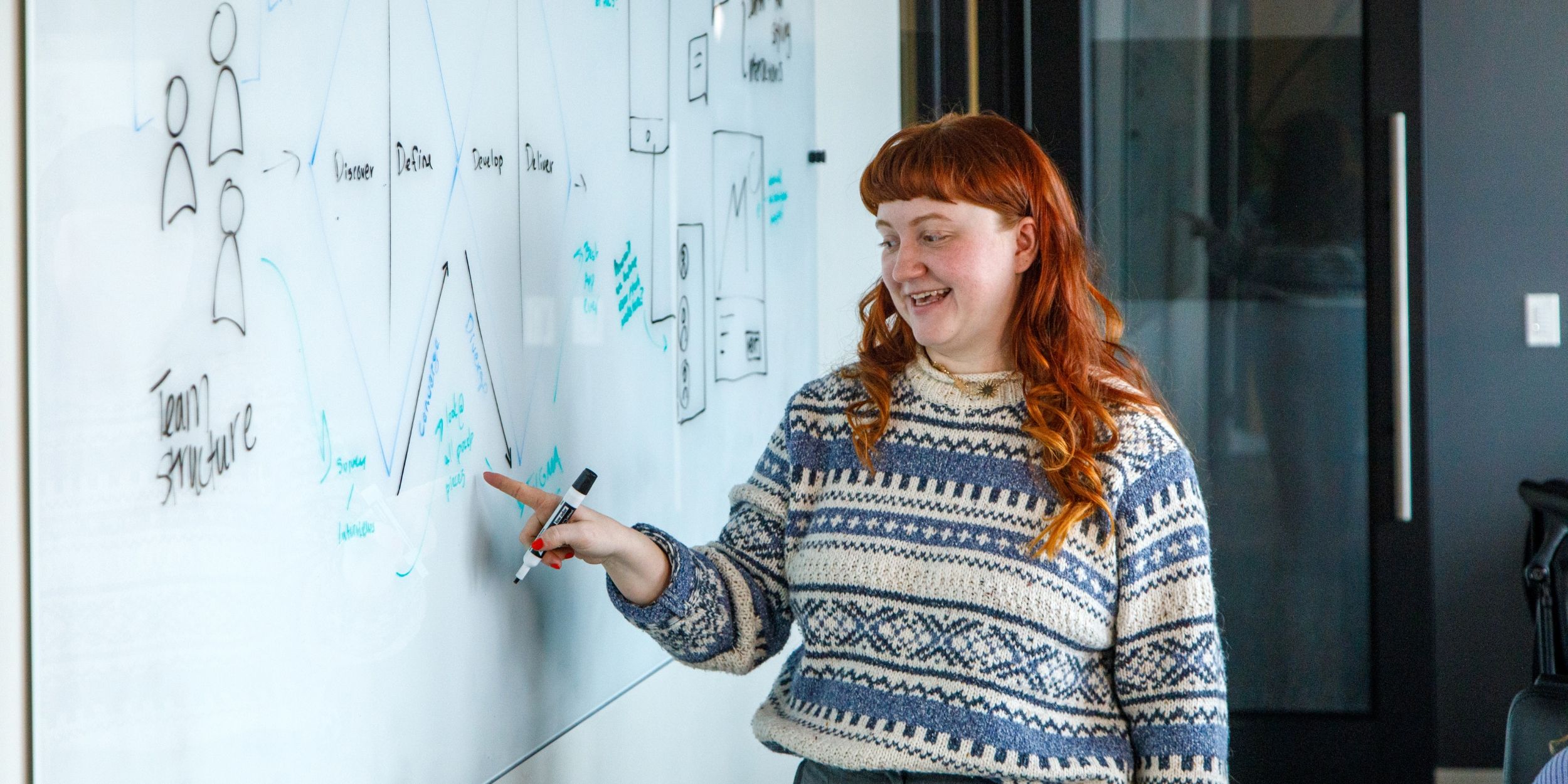
Product design, or UX design, is a strategic problem-solving process that leads to a valuable digital product. Learn what to expect when working with product designers.
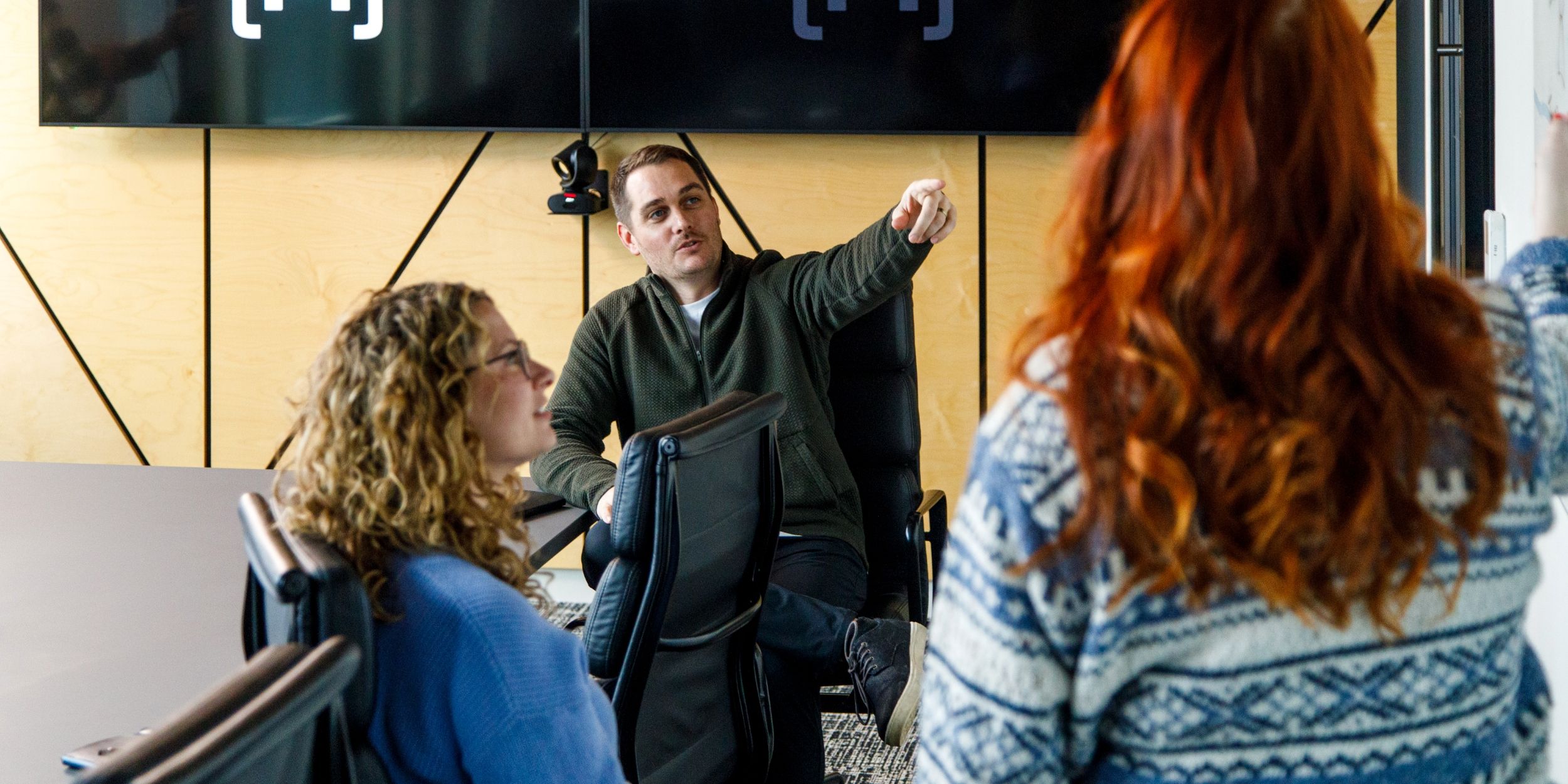
Achieve success in your custom software by starting with product strategy. Discover the key questions to ask to align your digital product with business goals and user needs.
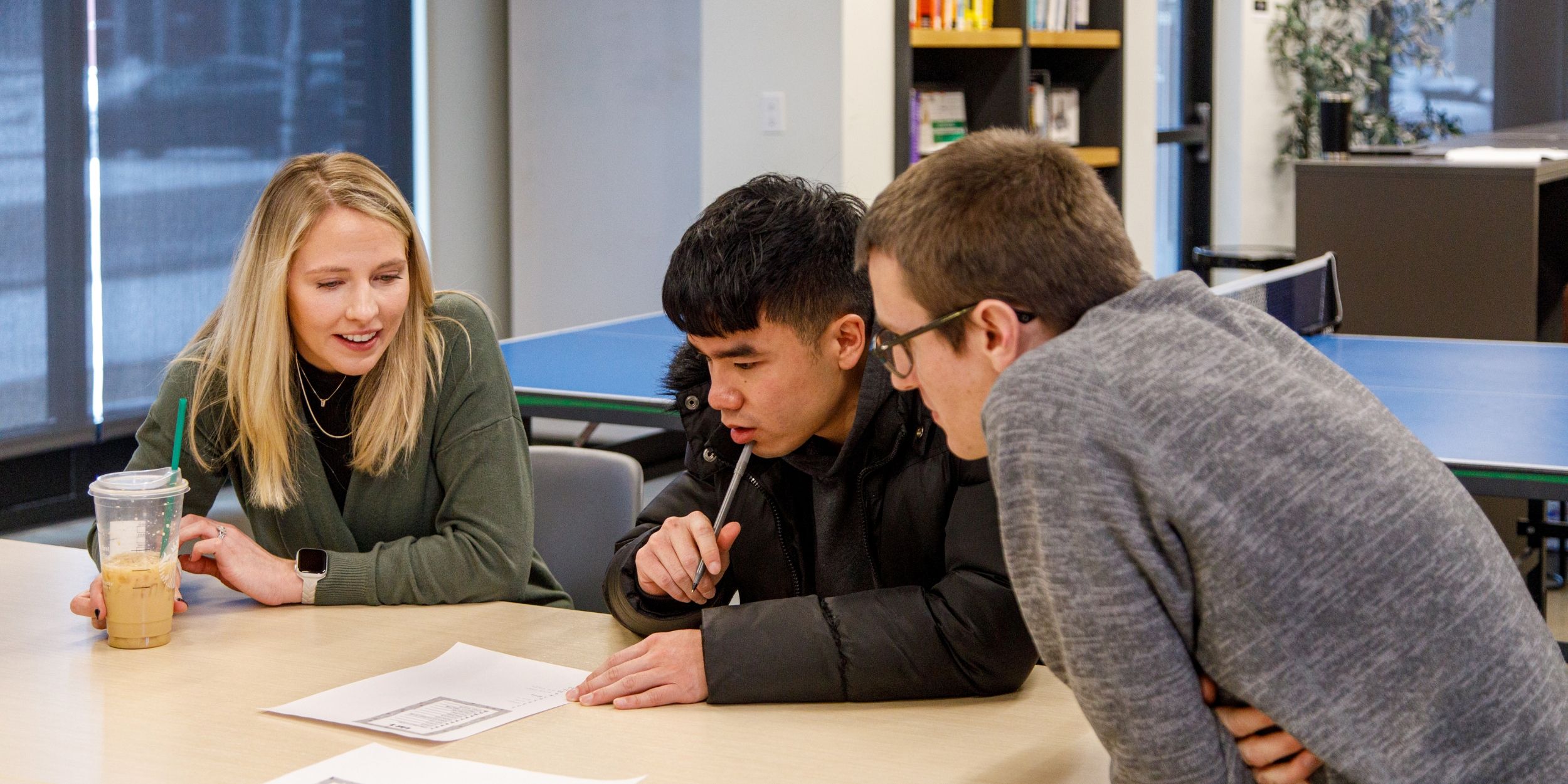
Delivery leads build great teams, provide excellent service to clients, and help MichiganLabs grow. Learn what you can expect when working with us!
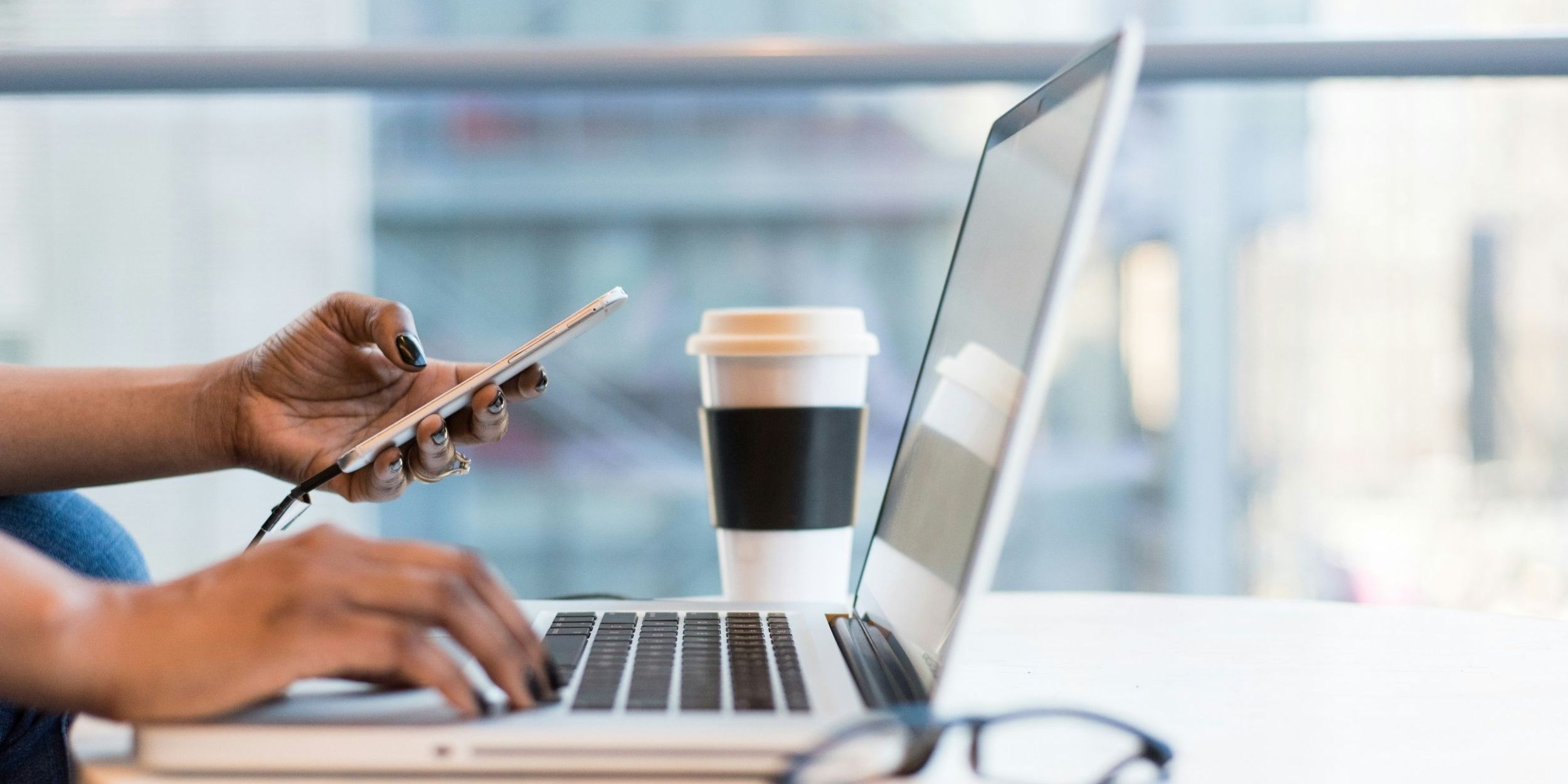
To decide between a web app and a mobile app, consider your target users, budget, functionality, and long-term goals. Discover key differences.
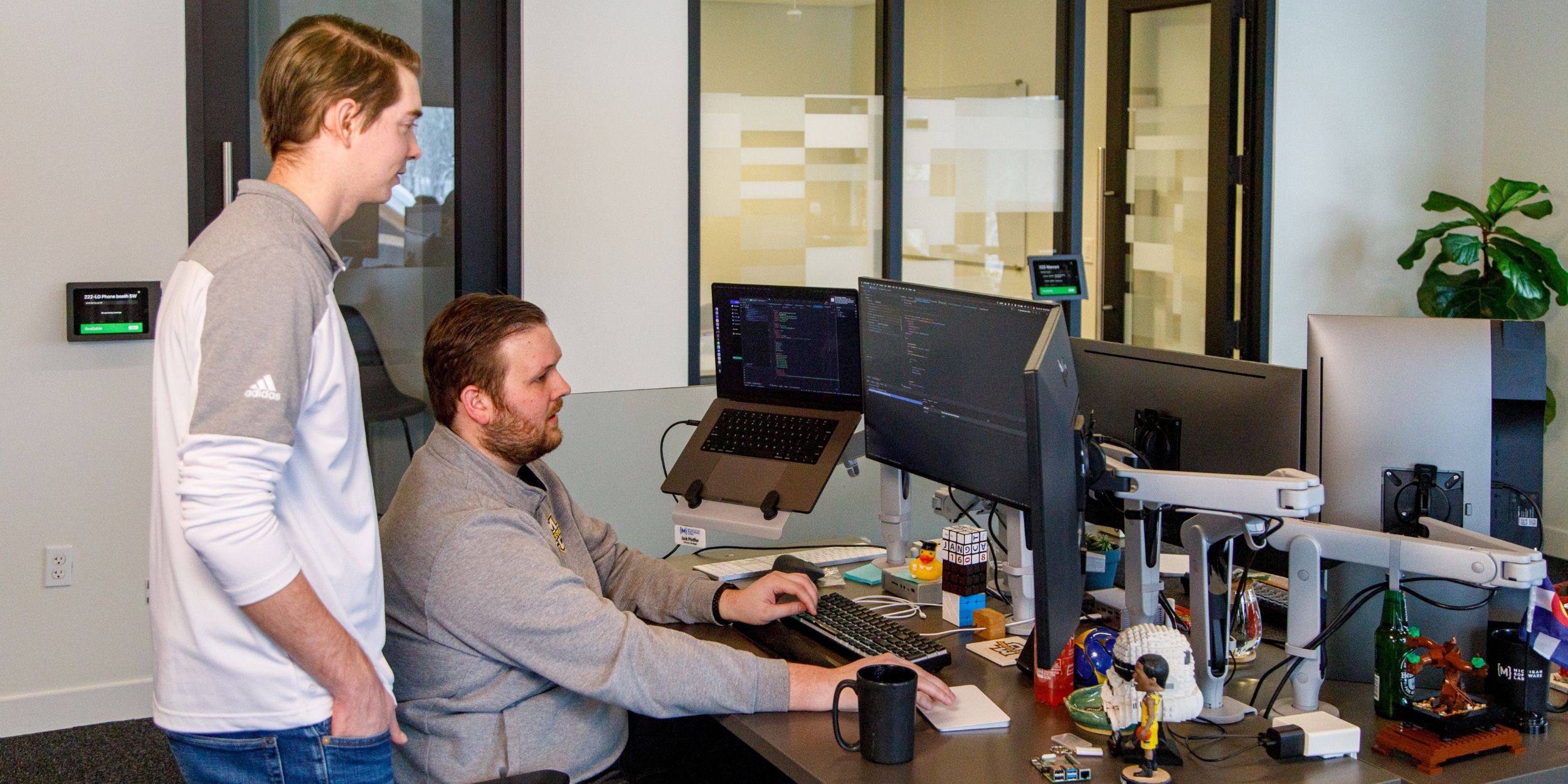
Developers leverage open source software to give benefits like faster time to market, increased security and support, and ease of future maintenance.
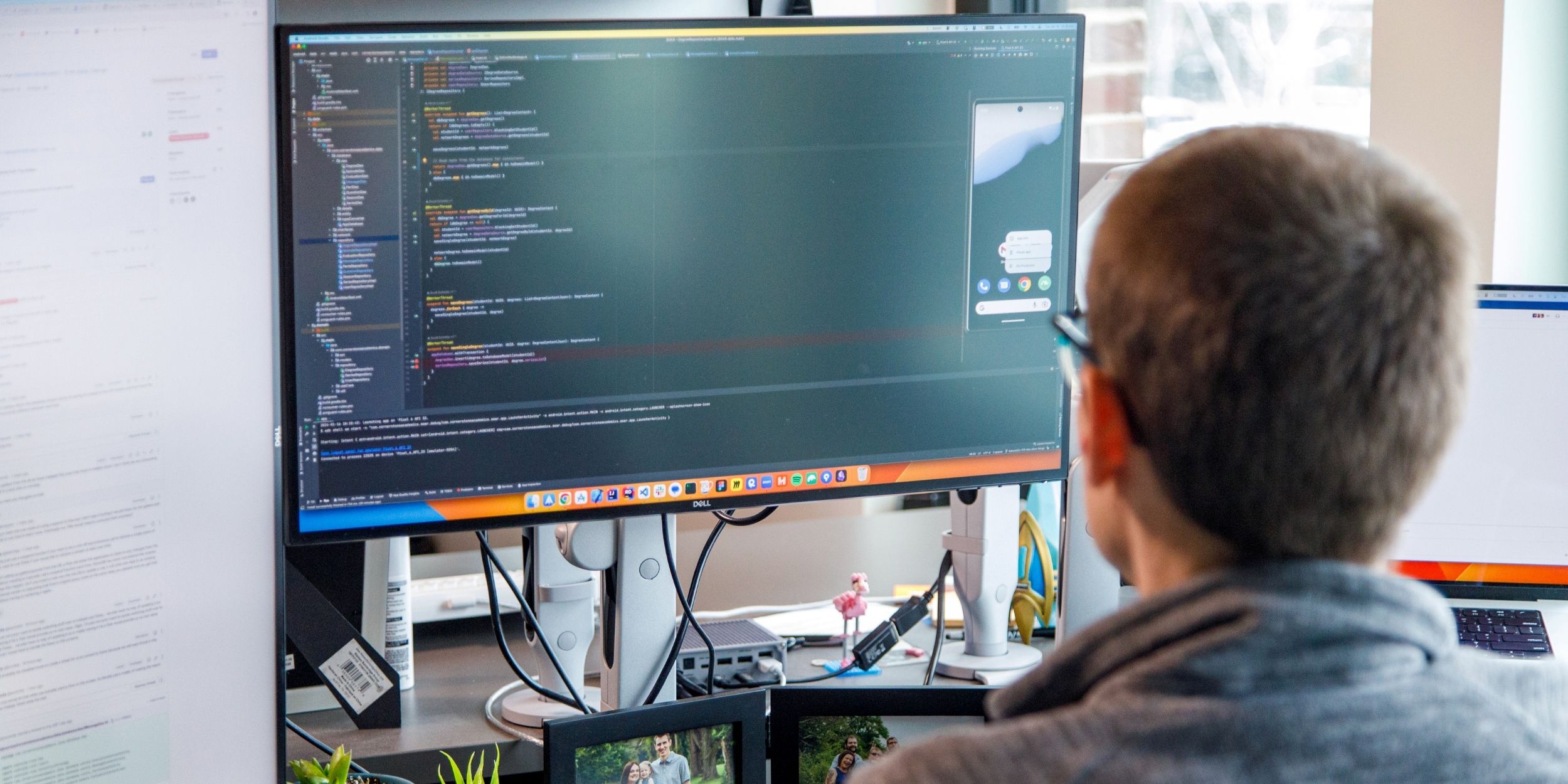
Legacy APIs are complex, often incompatible, and challenging to maintain. Our digital product consultants and developers share lessons learned.
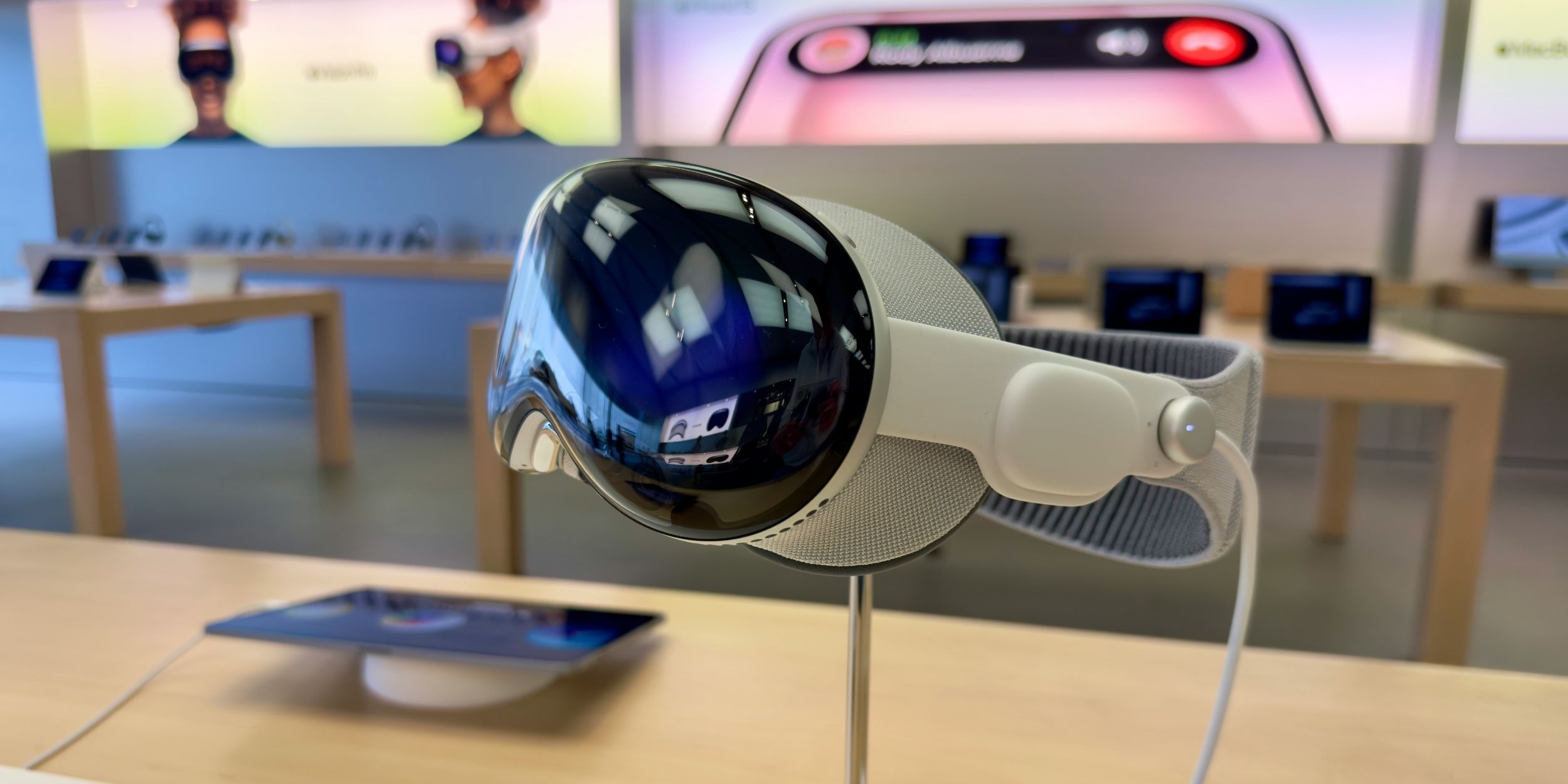
Common use cases for AR include education and training, product visualization, streamlined operations, and data visualization and analytics.
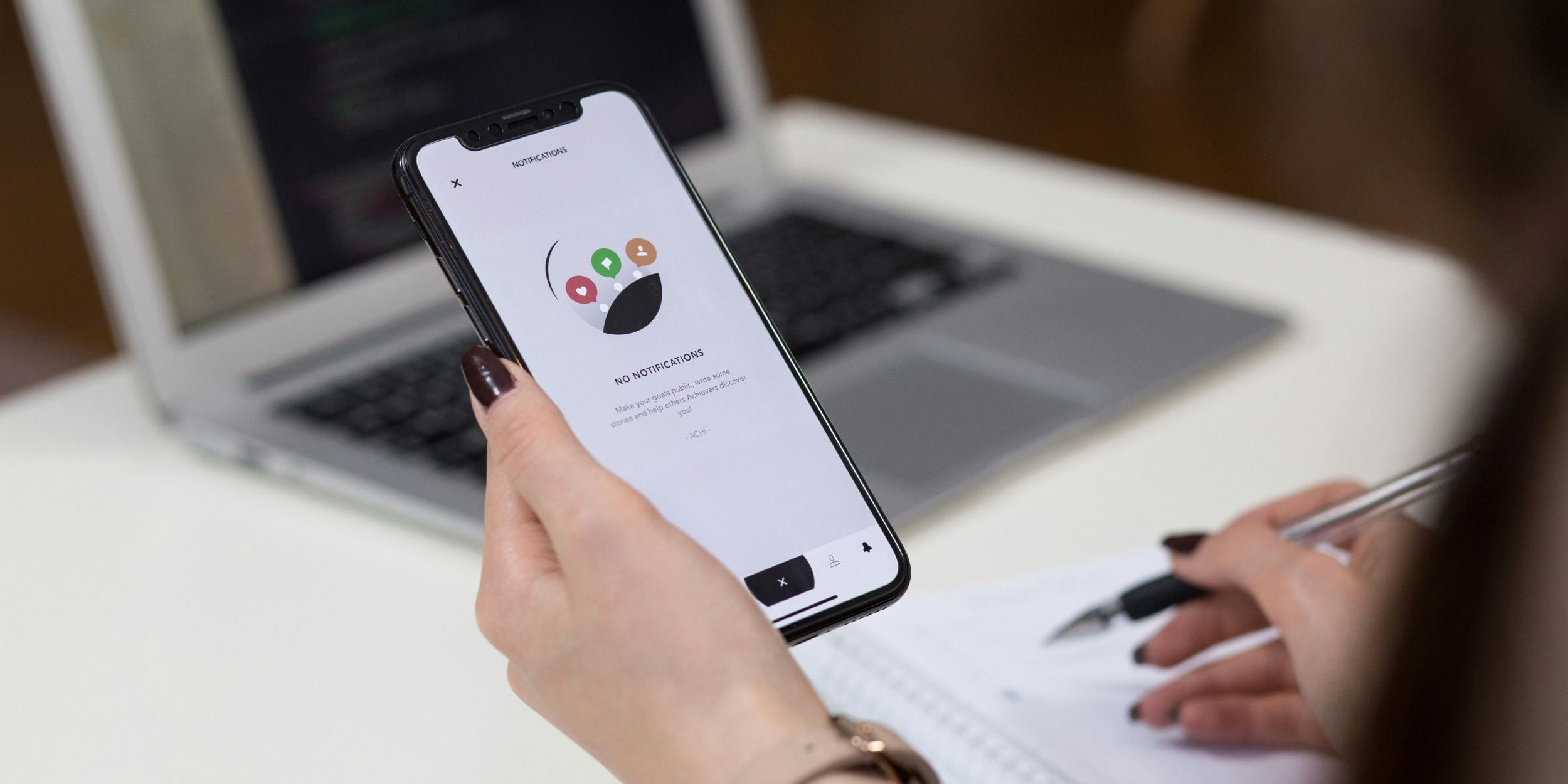
Discover why user research is crucial in software development. Learn effective methods to understand & meet user needs for successful digital products
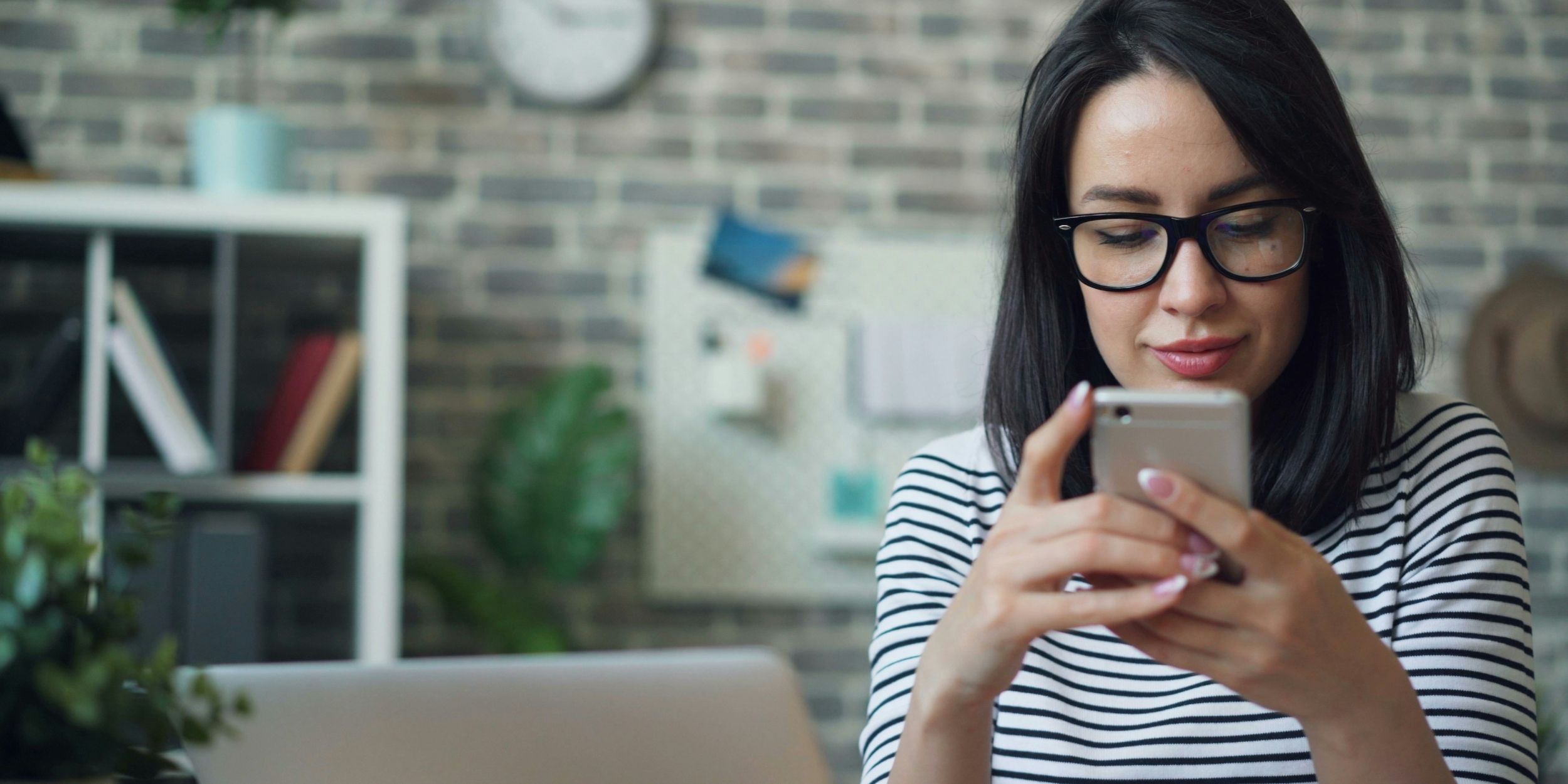
Focusing on value delivery—rather than just feature count—combines your business goals with your users’ needs to achieve real software ROI.
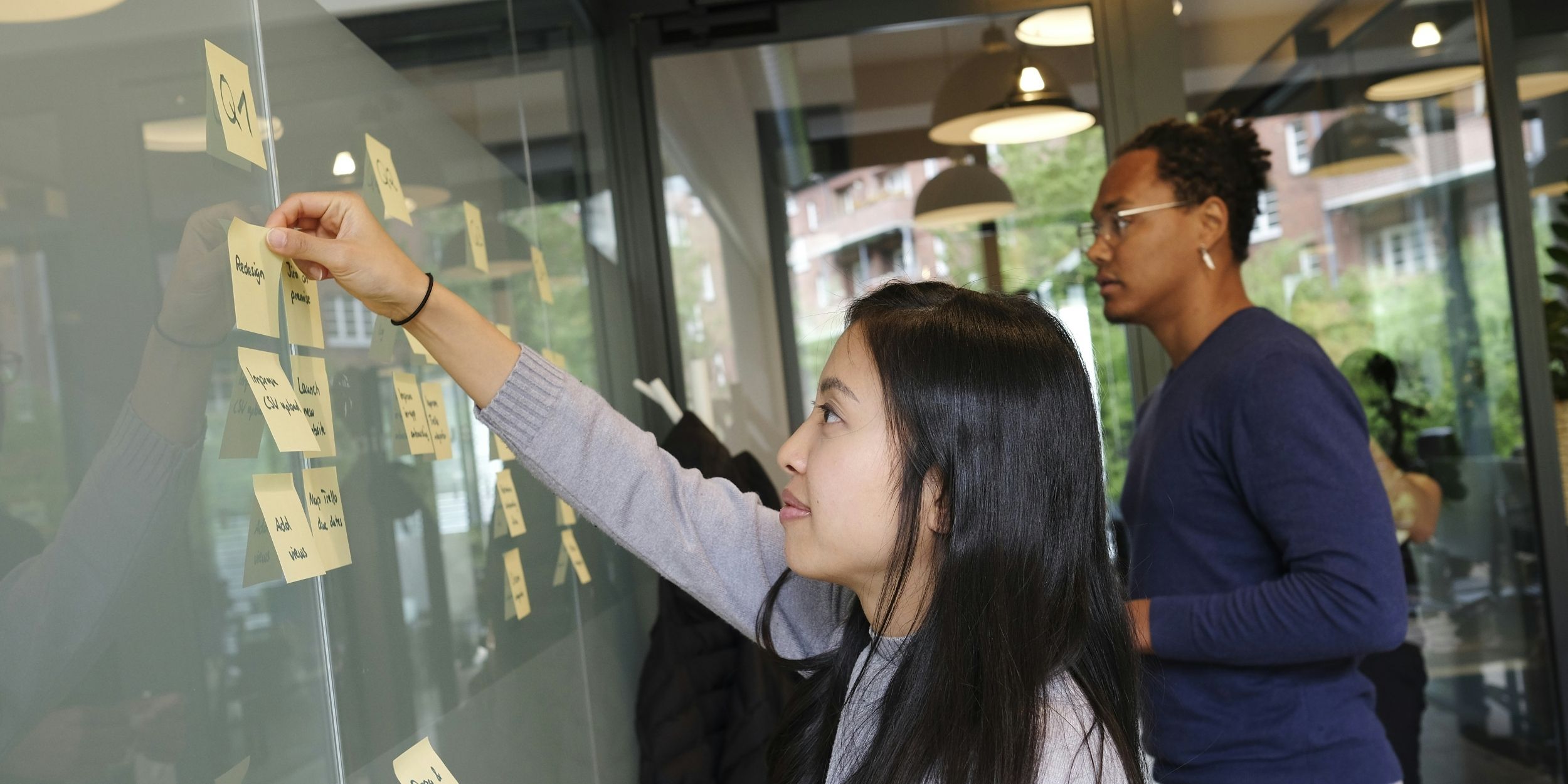
Product roadmaps are a strategic guide that outlines the development team’s intended direction. Learn how they help us make better decisions.
.jpg&w=3840&q=100)
Learn how MichiganLabs delivers fast software ROI by focusing on time to value, early releases, and key metrics for smarter, faster decision-making.
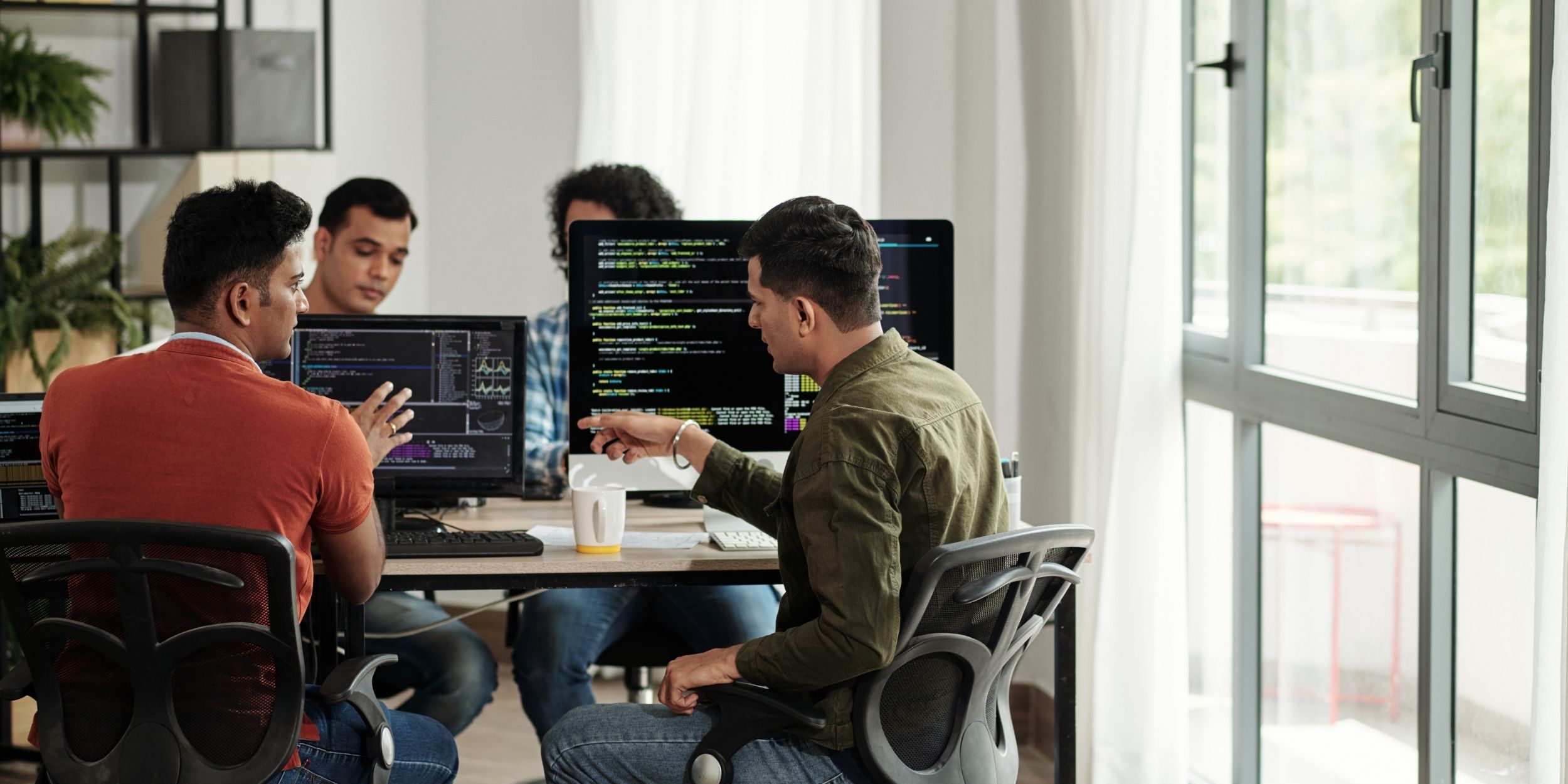
Optimize software ROI by making smart tech stack choices that boost performance, scalability, and cost-efficiency for long-term success
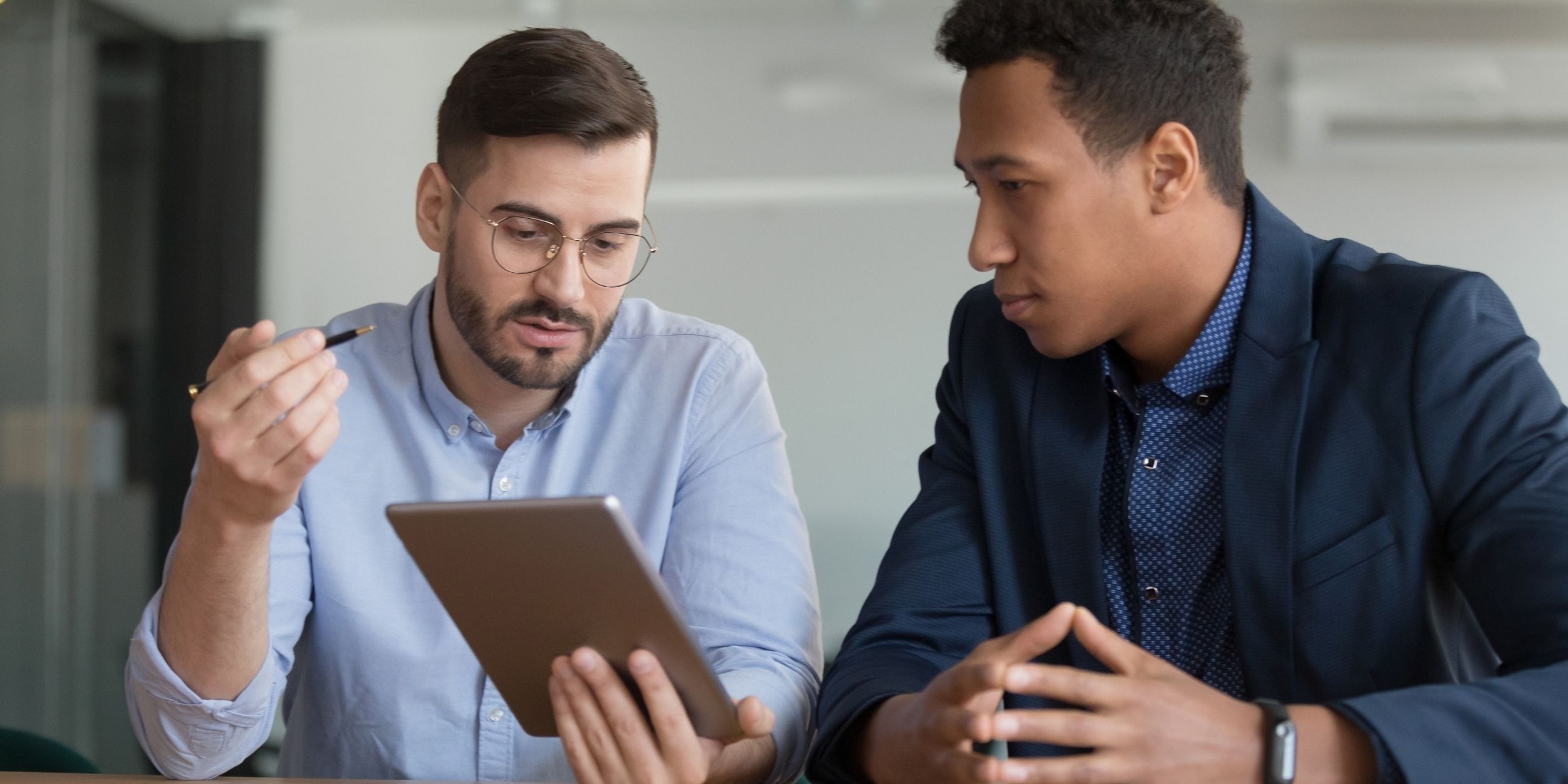
How to decide between building custom software, buying off-the-shelf, or using a hybrid solution. Explore key factors like cost and scalability.
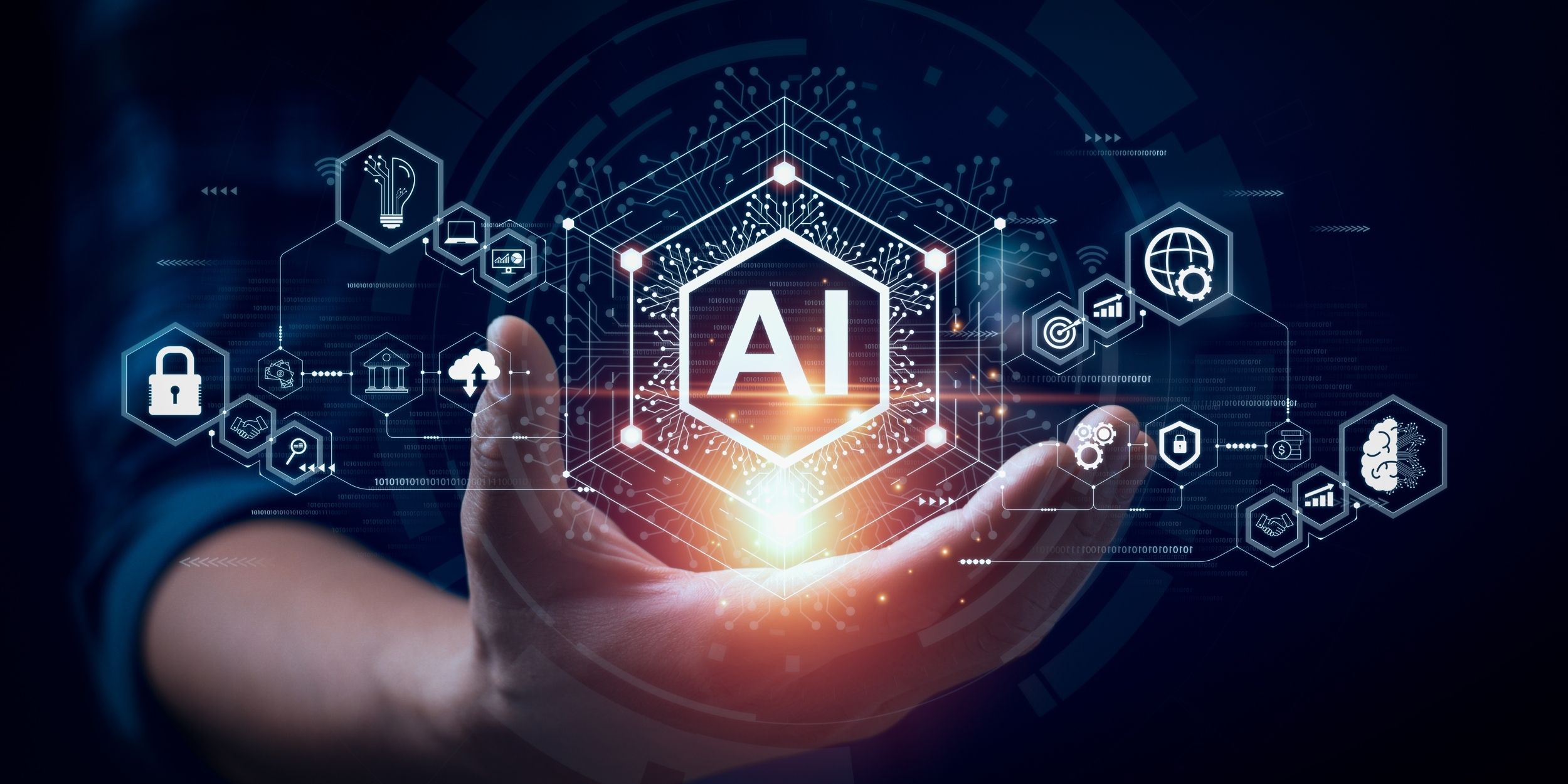
Explore the benefits and real-world applications of AI to improve efficiency, personalization, and decision-making for your business.